python tess文字识别
时间: 2023-09-26 12:15:02 浏览: 120
要使用Python进行文字识别,可以使用Tesseract库。首先,需要安装Tesseract以及相关的Python库。然后,可以使用以下步骤进行文字识别:
1. 导入所需的库:
```python
import pytesseract
from PIL import Image
```
2. 加载要识别的图片:
```python
img = Image.open("test.png")
```
3. 使用Tesseract进行文字识别:
```python
result = pytesseract.image_to_string(img)
```
4. 如果需要识别中文,可以添加`lang='chi_sim'`参数:
```python
result = pytesseract.image_to_string(img, lang='chi_sim')
```
5. 如果需要保存识别结果至文件中,可以使用以下代码:
```python
with open("result.txt", "w", encoding="utf-8") as file:
file.write(result)
```
请注意,以上步骤中的"test.png"应替换为您要识别的图片文件的路径。此外,您还需要确保已安装了Tesseract和相关依赖。
是一个示例代码,展示了如何使用Tesseract识别中文文本。
是另一个示例代码,展示了如何识别商品图片上的文字。
是使用命令行执行Tesseract的示例,将识别结果保存至文件中。
相关问题
python 截图识别
在Python中,截图识别通常涉及到计算机视觉(Computer Vision)领域的图像处理技术,特别是OCR(Optical Character Recognition,光学字符识别)部分。如果你想要识别屏幕上的文字,可以利用开源库如`pytesseract`,它是一个Python接口,用于访问Google的Tesseract OCR引擎。以下是一个简单的步骤:
1. 安装所需库:
```bash
pip install pytesseract opencv-python Pillow
```
2. 使用PIL(Pillow)读取截图并转换为适合OCR的格式:
```python
from PIL import ImageGrab
screenshot = ImageGrab.grab()
```
3. 将图片转为灰度,并优化为文本识别:
```python
gray_image = screenshot.convert('L')
tess_data_config = '--psm 6' # 设置识别模式(0-14),6表示单行文本
image_file = 'temp.png' # 临时保存截图
gray_image.save(image_file)
```
4. 调用Tesseract进行识别:
```python
import pytesseract
text = pytesseract.image_to_string(Image.open(image_file), lang='chi_sim') # 中文识别,lang可根据需要修改
```
5. 清理资源:
```python
os.remove(image_file)
```
阅读全文
相关推荐
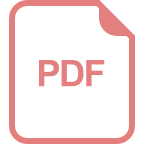
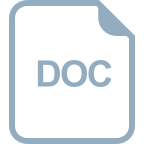
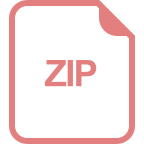
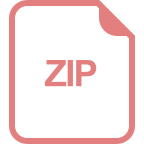
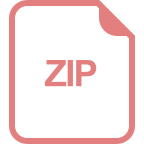
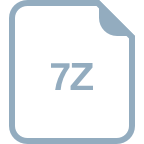
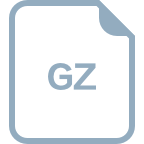
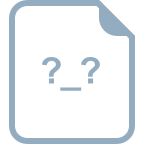
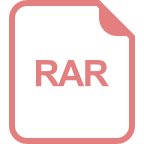
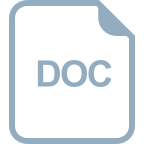
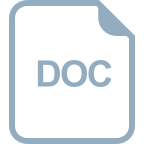
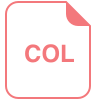
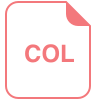
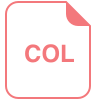
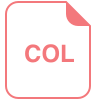
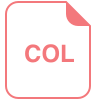