java多线程拷贝指定文件夹下的文件(不考虑子文件夹,只考虑当层文件夹下的文件),单个线程拷贝单个文件。原文件夹名、目标文件名由用户输入。所有线程完成工作后,输出“文件夹内文件拷贝完成”的消息
时间: 2024-10-13 13:16:49 浏览: 23
在Java中,你可以通过`java.util.concurrent.ExecutorService`和`java.io.File`类来实现这个功能。首先,你需要创建一个`CopyTask`类,该类包含一个具体的拷贝方法,它会接收源文件路径和目标文件路径作为参数。然后,你可以创建一个`CopyWorker`类,继承自`Thread`,并在run()方法中实例化并执行`CopyTask`。
```java
import java.io.File;
import java.io.IOException;
class CopyTask {
private String sourceFilePath;
private String targetFilePath;
public CopyTask(String source, String target) {
this.sourceFilePath = source;
this.targetFilePath = target;
}
public void copyFile() throws IOException {
File srcFile = new File(sourceFilePath);
File dstFile = new File(targetFilePath);
if (srcFile.exists()) {
try (InputStream in = new FileInputStream(srcFile);
OutputStream out = new FileOutputStream(dstFile)) {
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
System.out.println("Copied " + srcFile.getName() + " to " + dstFile.getAbsolutePath());
}
} else {
System.err.println("Source file not found: " + srcFile.getAbsolutePath());
}
}
}
class CopyWorker extends Thread {
private final CopyTask task;
public CopyWorker(CopyTask task) {
this.task = task;
}
@Override
public void run() {
try {
task.copyFile();
} catch (IOException e) {
System.err.println("Error copying file: " + e.getMessage());
}
}
}
public class Main {
public static void main(String[] args) {
// 获取用户输入的源目录和目标目录
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the source folder path: ");
String source = scanner.nextLine();
System.out.print("Enter the target folder path: ");
String target = scanner.nextLine();
// 创建CopyTasks列表
List<CopyTask> tasks = new ArrayList<>();
File folder = new File(source);
for (File file : folder.listFiles()) { // 只处理当前层级的文件
tasks.add(new CopyTask(file.getAbsolutePath(), target + "/" + file.getName()));
}
ExecutorService executor = Executors.newFixedThreadPool(tasks.size()); // 使用固定大小线程池
for (CopyTask task : tasks) {
executor.execute(task);
}
executor.shutdown(); // 关闭线程池
try {
executor.awaitTermination(Long.MAX_VALUE, TimeUnit.NANOSECONDS); // 等待所有任务完成
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
System.out.println("Files copied successfully.");
}
}
```
阅读全文
相关推荐
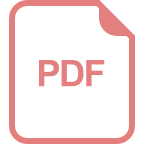
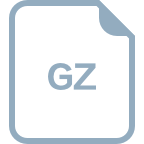
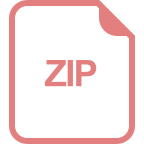















