c++设计一个person类,其属性包括name和id,其中name属性为指针,分别利用person类构造函数和拷贝构造函数建立对象,打印每个person类对象的信息。要求分别编写浅拷贝构造函数和深拷贝构造函数调试程序。
时间: 2024-12-08 19:18:16 浏览: 82
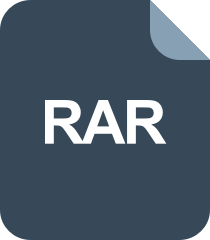
C++ 一般(普通)函数指针、类成员函数指针,用法区别,以及强制转换
在C++中,我们可以创建一个名为Person的类,包含两个成员变量name和id。为了实现浅拷贝构造函数和深拷贝构造函数,我们需要考虑指针的拷贝行为。下面是一个示例:
```cpp
#include <iostream>
#include <string>
class Person {
public:
// 成员变量
std::string* name;
int id;
// 构造函数
Person(const std::string& newName, int newId) : name(new std::string(newName)), id(newId) {} // 使用浅拷贝(默认行为)
// 浅拷贝构造函数(只复制指向的内存)
Person(const Person& other) : name(other.name), id(other.id) {}
// 深拷贝构造函数(完全复制所有内容)
Person(Person&& other) noexcept : name(other.name), id(other.id) { other.name = nullptr; other.id = 0; } // 使用移动语义
// 打印信息
void printInfo() const {
std::cout << "Name: " << (*name) << ", ID: " << id << std::endl;
}
private:
// 防止直接赋值(即禁止浅拷贝)
Person& operator=(const Person&) = delete;
};
int main() {
// 创建第一个Person对象
Person p1("Alice", 123);
// 打印p1的信息
p1.printInfo();
// 使用浅拷贝构造函数创建第二个对象(共享引用)
Person p2(p1); // 注意这里并没有创建新的字符串对象,而是共享了p1的name指针
// 打印p2的信息
p2.printInfo();
// 使用深拷贝构造函数创建第三个对象(独立副本)
Person p3(p1); // 这里会创建一个新的std::string对象,并复制p1的id
// 打印p3的信息
p3.printInfo();
return 0;
}
```
在这个例子中,`main()`函数中展示了如何使用不同构造函数创建对象。注意,浅拷贝构造函数仅复制指针本身,如果name是一个复杂的对象,可能会引发问题;而深拷贝构造函数则会复制整个对象,避免这些问题。同时,我们还删除了浅拷贝赋值运算符,以防止意外的浅拷贝。
阅读全文
相关推荐
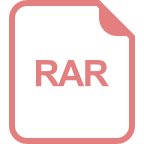
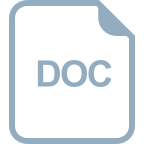








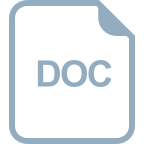
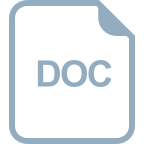
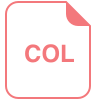
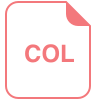



