平年一年中的12个月,1、3、5、7、8、10、12月是31天,4、6、9、11月是30天,2月是28天,用C语言实现在控制台上任意输入一个月份,就可以知道这个月有多少天。
时间: 2024-09-25 11:01:46 浏览: 43
你可以创建一个简单的C程序来实现这个功能。我们可以使用条件语句根据输入的月份来判断每个月的天数。以下是一个基本的示例:
```c
#include <stdio.h>
int main() {
int month, days;
// 获取用户的输入
printf("Enter the month (1-12): ");
scanf("%d", &month);
switch (month) {
case 1: // January
case 3: // March
case 5: // May
case 7: // July
case 8: // August
case 10: // October
case 12: // December
days = 31;
break;
case 4: // April
case 6: // June
case 9: // September
case 11: // November
days = 30;
break;
case 2: // February
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)) { // Leap year
days = 29;
} else {
days = 28;
}
break;
default: // Invalid input
printf("Invalid month. Please enter a number between 1 and 12.\n");
return 1; // Exit with error code
}
printf("The month %d has %d days.\n", month, days);
return 0;
}
```
在这个程序中,我们假设用户输入的是公历的年份(没有考虑到世纪闰年),所以对于二月,如果这一年能被4整除并且不能被100整除,或者能被400整除,那么它就是闰年,有29天。
如果你需要处理用户输入的年份,请添加对闰年的检查逻辑,并相应地更新二月的天数计算。此外,为了简化输入验证,这里直接要求用户输入1到12的数字,如果输入其他值,程序会提示错误并退出。
阅读全文
相关推荐
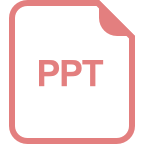
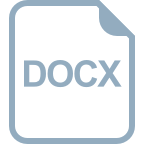
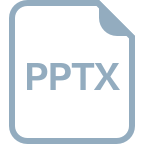















