设有向图G采用邻接表存储,设计算法求图G中每个顶点的入度。C++完整代码实现
时间: 2024-12-15 15:21:28 浏览: 33
在C++中,你可以使用邻接表存储图并遍历它来计算每个顶点的入度。首先,你需要创建一个邻接表数据结构,然后从每个顶点开始,遍历其邻居列表并递增对应的入度计数。以下是基本的代码实现:
```cpp
#include <iostream>
#include <unordered_map>
// 定义邻接表节点
struct AdjListNode {
int vertex;
std::vector<AdjListNode*> neighbors;
};
// 图类
class Graph {
private:
std::unordered_map<int, AdjListNode*> nodes; // 使用哈希表存储顶点及其邻接节点
public:
void addEdge(int src, int dest) {
if (nodes.find(src) == nodes.end()) {
nodes[src] = new AdjListNode{src};
}
nodes[src]->neighbors.push_back(&nodes[dest]);
}
void computeInDegrees() {
for (const auto& node : nodes) {
int vertex = node.first;
int inDegree = 0;
// 遍历邻居并增加入度
for (AdjListNode* neighbor : node.second.neighbors) {
++inDegree;
}
std::cout << "Vertex " << vertex << " has an in-degree of " << inDegree << std::endl;
}
}
};
int main() {
Graph graph;
// 添加边到图中...
graph.addEdge(1, 2);
graph.addEdge(2, 3);
graph.addEdge(3, 4);
graph.addEdge(1, 4);
graph.computeInDegrees();
return 0;
}
```
在这个代码中,`Graph` 类有一个 `nodes` 字典,键是顶点ID,值是包含该顶点所有邻居指针的链表。`computeInDegrees` 函数通过遍历每个节点和它的邻居,统计出度。注意,在实际应用中,需要处理已存在的边,并确保在添加边时更新邻接表。
阅读全文
相关推荐
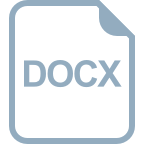
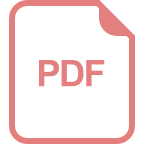
















