Java中解压zip文件到另一个目录中的示例代码
时间: 2024-09-07 14:06:27 浏览: 40
在Java中,你可以使用`java.util.zip`包中的`ZipInputStream`和`ZipOutputStream`来进行zip文件的解压缩。下面是一个基本的示例,它解压一个zip文件到指定的目标目录:
```java
import java.io.*;
import java.util.Enumeration;
import java.util.zip.*;
public class UnzipExample {
public static void main(String[] args) {
String sourceZip = "/path/to/source.zip";
String destinationDir = "/path/to/destination/";
try {
// 创建目标目录(如果不存在)
File destFolder = new File(destinationDir);
if (!destFolder.exists()) {
destFolder.mkdirs();
}
// 创建输入和输出流
FileInputStream fis = new FileInputStream(sourceZip);
ZipInputStream zis = new ZipInputStream(fis);
ZipEntry entry;
while ((entry = zis.getNextEntry()) != null) {
// 检查是否是目录
if (!entry.isDirectory()) {
// 获取输出文件路径
String filePathInDest = destinationDir + entry.getName();
// 创建目标文件
FileOutputStream fos = new FileOutputStream(filePathInDest);
// 解压内容到目标文件
byte[] buffer = new byte[1024];
int length;
while ((length = zis.read(buffer)) >= 0) {
fos.write(buffer, 0, length);
}
// 关闭流
fos.close();
}
// 关闭当前条目的输入流
zis.closeEntry();
}
// 关闭zip输入流
zis.close();
System.out.println("Unzipping complete!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这个示例假设源zip文件已经存在,并且目标目录可以访问。运行这个程序后,源zip文件的内容会被解压到指定的`destinationDir`下。
阅读全文
相关推荐
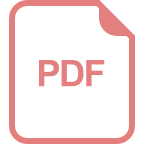
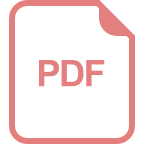
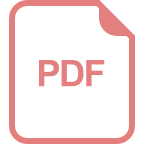















