TLV各种类型数据组包C语言
时间: 2024-09-14 16:00:19 浏览: 38
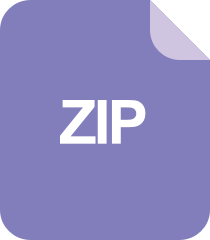
msp430单片机_DAC-TLV5617_c语言

TLV (Type-Length-Value) 是一种数据封装格式,在很多通信协议和数据结构中广泛使用,特别是网络、配置文件和数据存储等领域。C语言中处理TLV数据通常涉及以下几个步骤:
1. 定义结构:在C语言里,你可以创建一个结构体,包含三个字段:type(类型标识)、length(长度)和value(值)。例如:
```c
typedef struct {
uint8_t type;
uint16_t length;
void* value; // 指向实际数据的位置
} TlvEntry;
```
2. 编码:对于不同类型的数据,你可以编写函数来将它们编码成TLV格式。比如添加整数、字符串或自定义结构体到`TlvEntry`中。
```c
void encode_int(TlvEntry* entry, int value) {
entry->type = ...; // 根据需要设置类型
entry->length = htons(sizeof(int)); // 高16位表示长度,使用htons转换网络字节序
entry->value = &((uint16_t*)entry->value)[0]; // 存储int的地址
*((uint16_t*)entry->value) = htonl(value); // 转换并存储值
}
void encode_string(TlvEntry* entry, const char* str) {
entry->type = ...;
entry->length = htons(strlen(str) + 1); // 包含NULL终止符
entry->value = ...; // 存储字符串的地址
memcpy(entry->value, str, strlen(str));
}
```
3. 解码:从接收到的TLV数据中,可以遍历每个条目并提取信息。
```c
void decode_tlv(const TlvEntry* entries, size_t count) {
for (size_t i = 0; i < count; i++) {
switch (entries[i].type) {
case TYPE_INTEGER:
printf("Integer: %d\n", ntohl(*((uint16_t*)entries[i].value)));
break;
case TYPE_STRING:
printf("String: %s\n", (char*)entries[i].value);
break;
// 其他类型处理...
}
}
}
```
阅读全文
相关推荐
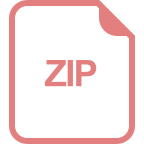
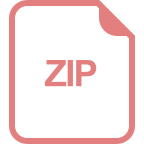
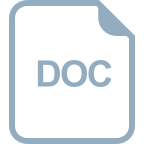
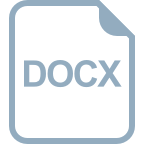
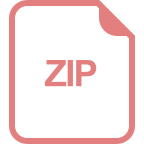
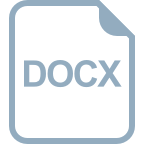
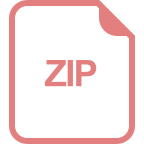
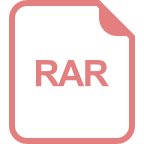
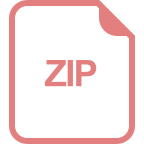
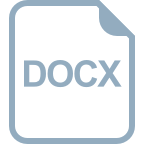
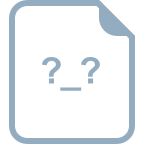
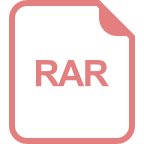
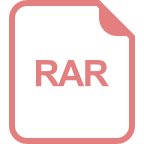
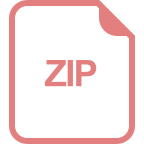