java中写入文件时如何设置每12秒写入一次
时间: 2024-11-18 20:15:52 浏览: 6
在Java中,如果你想要每12秒写入一次文件,通常我们会结合定时任务(如`ScheduledExecutorService`)与IO操作来实现。这里不是一个完整的代码示例,但可以提供一个思路:
```java
import java.io.FileWriter;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class FileWritingTask {
private static final ScheduledExecutorService executor = Executors.newSingleThreadScheduledExecutor();
public static void main(String[] args) {
String contentToWrite = "Your string to write";
Path filePath = Paths.get("your_file_path.txt");
// 每12秒执行写入任务
executor.scheduleAtFixedRate(() -> {
try {
if (Files.exists(filePath)) {
Files.append(filePath, contentToWrite.getBytes(), StandardOpenOption.APPEND);
} else {
Files.write(filePath, contentToWrite.getBytes());
}
} catch (IOException e) {
System.err.println("Error writing to file: " + e.getMessage());
}
}, 0, 12, TimeUnit.SECONDS);
// 当程序结束时关闭执行器以取消所有未来计划的任务
Runtime.getRuntime().addShutdownHook(new Thread(() -> executor.shutdown()));
}
}
```
这段代码创建了一个单线程定时任务,它每12秒会尝试写入指定的内容到文件。如果文件已存在,它将在文件末尾追加新的内容;如果文件不存在,则创建一个新的文件并写入内容。
注意:这个例子假设你的应用程序有权限写入给定的文件路径。另外,你需要确保你的环境支持`java.nio.file`包,它是Java 7及以上版本的标准库。
阅读全文
相关推荐
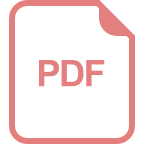
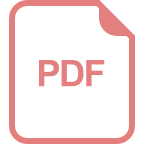
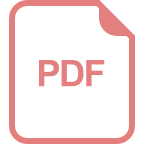















