编写一个结构体使得用结构体存放所有数据,每个功能都用函数实现,输入十个学生的学号和三门课的成绩计算每个学生的总分
时间: 2024-09-29 16:02:16 浏览: 15
为了实现这个需求,我们可以定义一个名为`Student`的结构体,它包含学号、姓名以及三门课程的成绩数组。同时,我们编写几个相关的函数:初始化学生信息、添加成绩和计算总分。以下是使用Go语言的一个示例:
```go
package main
type Student struct {
ID int
Name string
Scores [3]int // 存储三门课程的成绩
}
// 初始化一个新的学生
func NewStudent(id int, name string) *Student {
return &Student{ID: id, Name: name, Scores: [3]int{}}
}
// 添加成绩到学生记录
func (s *Student) AddScore(course, score int) {
s.Scores[course - 1] = score
}
// 计算学生总分
func (s *Student) CalculateTotal() int {
total := 0
for _, score := range s.Scores {
if score > 0 { // 可以加上检查分数是否有效的逻辑
total += score
}
}
return total
}
func main() {
student := NewStudent(1, "张三")
student.AddScore(1, 90)
student.AddScore(2, 85)
student.AddScore(3, 95)
total := student.CalculateTotal()
fmt.Printf("学生 %d 的总分为:%d\n", student.ID, total)
// 同样的过程可以为其他9名学生重复
}
```
在这个例子中,`NewStudent`用于创建新的学生,`AddScore`用于添加成绩,而`CalculateTotal`则计算并返回学生的总分。在`main`函数中展示了如何使用这些函数。
相关推荐
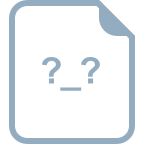
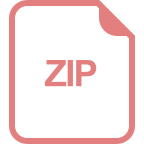
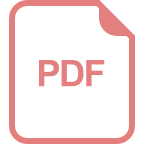















