请输入自己的姓名与学号,输入3门课的成绩,计算平均成绩后显示在控制台中。如果学号的尾数为奇数,那么还需在控制台上显示最高成绩;如果自己的学号尾数为偶数,那么需要在控制台上显示最低成绩。
时间: 2024-10-17 19:10:14 浏览: 25
这是一个简单的编程练习,通常用于教授控制台应用程序的基本操作。假设我们有一个名为`Student`的类,包含姓名、学号和成绩属性,我们可以这样做:
```java
class Student {
String name;
int studentId;
double[] scores;
public Student(String name, int studentId, double[] scores) {
this.name = name;
this.studentId = studentId;
this.scores = scores;
}
// 计算平均成绩
double calculateAverage() {
double sum = 0;
for (double score : scores) {
sum += score;
}
return sum / scores.length;
}
}
public class Main {
public static void main(String[] args) {
String name = "张三";
int studentId = 1234; // 假设尾数为偶数
double[] scores = {85, 92, 98}; // 三门课程成绩
Student student = new Student(name, studentId, scores);
double averageScore = student.calculateAverage();
System.out.println("学生姓名:" + name);
System.out.println("平均成绩:" + averageScore);
if (studentId % 2 == 1) { // 判断尾数是否为奇数
System.out.println("最高成绩:" + Collections.max(scores));
} else {
System.out.println("最低成绩:" + Collections.min(scores));
}
}
}
```
在这个例子中,我们首先创建了一个`Student`对象,然后调用它的`calculateAverage`方法获取平均成绩。接着,根据学生的学号尾数决定是否显示最高或最低成绩。
阅读全文
相关推荐








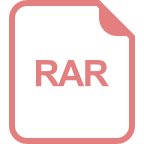




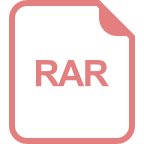




