c++定义单链表的类,实现一个带头结点的单链表的逆置,并将逆置前后的单链表分别遍历输出。单链表中各结点的值和结点个数等自定。
时间: 2024-10-10 21:10:30 浏览: 34
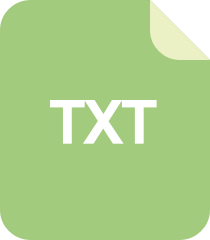
单链表的各种操作实现
在C++中,我们可以定义一个`Node`类表示链表节点,包含一个数据成员和指向下一个节点的指针。然后定义一个`SingleLinkedList`类,其中包含头节点、插入节点的功能以及链表的反转操作。下面是一个简单的实现:
```cpp
#include <iostream>
// 定义链表节点
class Node {
public:
int data;
Node* next;
Node(int val) : data(val), next(nullptr) {}
};
// 单链表类
class SingleLinkedList {
private:
Node* head; // 头节点
public:
SingleLinkedList() : head(nullptr) {}
// 插入节点到链表尾部
void insert(int value) {
if (!head) {
head = new Node(value);
} else {
Node* current = head;
while (current->next) {
current = current->next;
}
current->next = new Node(value);
}
}
// 链表反转
void reverse() {
Node* prev = nullptr;
Node* current = head;
Node* nextTemp;
while (current) {
nextTemp = current->next;
current->next = prev;
prev = current;
current = nextTemp;
}
head = prev;
}
// 遍历并打印链表
void printList() {
Node* temp = head;
while (temp) {
std::cout << temp->data << " ";
temp = temp->next;
}
std::cout << "\n";
}
// 打印反转后的链表
void printReversedList() {
Node* temp = head;
while (temp) {
std::cout << temp->data << " ";
temp = temp->next;
}
std::cout << "\nReversed: ";
temp = head;
while (temp) {
std::cout << temp->data << " ";
temp = temp->next;
}
std::cout << "\n";
}
};
```
现在你可以创建一个`SingleLinkedList`实例,插入一些节点,然后反转链表并打印原始链表和反转后的链表。
阅读全文
相关推荐
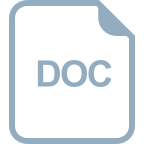
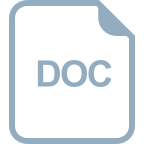


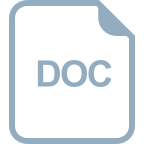












