webservice+json
时间: 2023-11-18 07:04:10 浏览: 51
WebService是一种基于Web的远程调用技术,它使用XML格式进行数据交互。而JSON是一种轻量级的数据交换格式,比XML更加简洁和易于解析。因此,WebService和JSON的结合可以实现跨平台、跨语言的数据交互。
下面是一个使用Java中的net.sf.json库实现WebService+JSON的例子:
```java
// 定义一个WebService接口
@WebService
public interface HelloWorld {
@WebMethod
String sayHello(String name);
}
// 实现WebService接口
@WebService(endpointInterface = "com.example.HelloWorld")
public class HelloWorldImpl implements HelloWorld {
public String sayHello(String name) {
return "Hello " + name + "!";
}
}
// 发布WebService服务
public class WebServicePublisher {
public static void main(String[] args) {
String url = "http://localhost:8080/HelloWorld";
Endpoint.publish(url, new HelloWorldImpl());
System.out.println("WebService服务已发布:" + url);
}
}
// 使用JSON格式调用WebService服务
public class WebServiceClient {
public static void main(String[] args) {
String url = "http://localhost:8080/HelloWorld";
String name = "Alice";
JSONObject request = new JSONObject();
request.put("name", name);
String response = HttpClientUtil.doPost(url, request.toString());
JSONObject result = JSONObject.fromObject(response);
String message = result.getString("message");
System.out.println(message); // 输出:Hello Alice!
}
}
```
上述代码中,我们定义了一个简单的HelloWorld接口和实现类,并使用Endpoint.publish()方法将其发布为WebService服务。然后,我们使用net.sf.json库将请求数据和响应数据转换为JSON格式,并使用HttpClientUtil.doPost()方法发送POST请求调用WebService服务。最后,我们将响应数据解析为JSON格式,并获取其中的message字段作为调用结果。
相关推荐
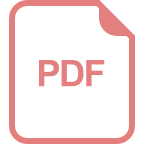














