将Java的关键字保存在文本文件中,判断一个字符串是否为Java的关键字。 编写java代码
时间: 2024-12-19 13:24:40 浏览: 6
在Java中,你可以创建一个文本文件(通常是`.txt`格式),其中包含所有的关键字列表,然后编写一个程序来读取这个文件并检查给定的字符串是否在这个列表中。以下是一个简单的示例:
首先,你需要创建一个包含所有Java关键字的文本文件(例如`keywords.txt`):
```
abstract
assert
boolean
break
byte
case
catch
char
class
const
continue
default
do
double
else
enum
extends
final
finally
float
for
goto
if
implements
import
instanceof
int
interface
long
native
new
package
private
protected
public
return
short
static
strictfp
super
switch
synchronized
this
throw
throws
transient
try
void
volatile
while
true
false
null
```
然后,你可以使用Java的文件I/O和集合库来实现这个功能:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.util.HashSet;
public class KeywordChecker {
private static final String KEYWORDS_FILE = "keywords.txt";
public static boolean isKeyword(String str) {
HashSet<String> keywords = loadKeywords();
return keywords.contains(str);
}
private static HashSet<String> loadKeywords() {
HashSet<String> result = new HashSet<>();
try (BufferedReader reader = new BufferedReader(new FileReader(KEYWORDS_FILE))) {
String line;
while ((line = reader.readLine()) != null) {
result.add(line.trim());
}
} catch (Exception e) {
System.err.println("Error loading keywords: " + e.getMessage());
}
return result;
}
public static void main(String[] args) {
String testString = "public";
if (isKeyword(testString)) {
System.out.println(testString + " is a Java keyword.");
} else {
System.out.println(testString + " is not a Java keyword.");
}
}
}
```
在这个代码中,`loadKeywords()`函数用于从文件中加载关键字,`isKeyword()`函数则检查输入的字符串是否在这些关键字中。
运行`main`方法,传入一个字符串即可测试。
阅读全文
相关推荐
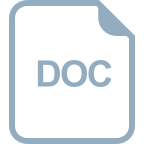
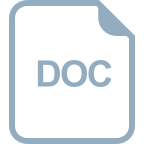
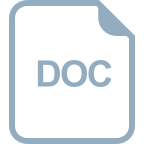

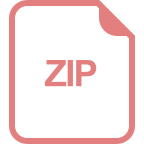
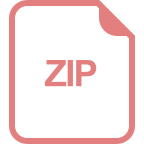
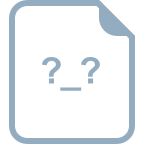
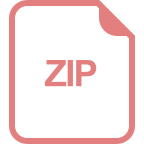
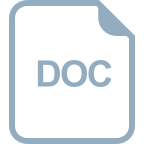

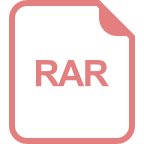
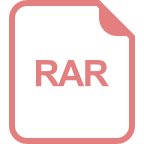
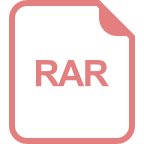
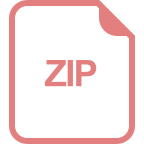
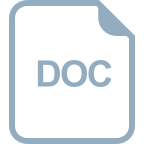
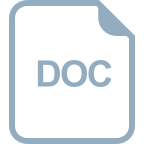
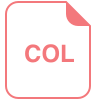
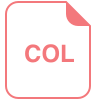
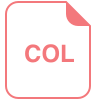