java await()
时间: 2023-09-08 08:12:39 浏览: 123
`await()` 方法是在 Java 的多线程编程中使用的一个方法,它用于使当前线程等待其他线程完成执行。
在 Java 中,可以通过 `Thread` 类的 `join()` 方法来实现线程的等待,但是 `join()` 方法只能等待单个线程的完成。而 `await()` 方法则是在 `java.util.concurrent` 包中的 `CountDownLatch`、`CyclicBarrier` 和 `Semaphore` 等类中使用的,可以等待多个线程的完成。
以 `CountDownLatch` 为例,它是一个同步辅助类,可以使一个或多个线程等待其他线程完成操作后再继续执行。通过 `CountDownLatch` 的 `await()` 方法可以使当前线程进入等待状态,直到计数器变为零。当其他线程完成任务后,可以通过 `CountDownLatch` 的 `countDown()` 方法将计数器减一,直到计数器变为零后,所有等待的线程将被唤醒继续执行。
下面是使用 `CountDownLatch` 的示例代码:
```java
import java.util.concurrent.CountDownLatch;
public class AwaitExample {
public static void main(String[] args) throws InterruptedException {
CountDownLatch latch = new CountDownLatch(3); // 创建一个计数器初始值为3
// 创建三个线程
Thread thread1 = new WorkerThread(latch);
Thread thread2 = new WorkerThread(latch);
Thread thread3 = new WorkerThread(latch);
// 启动三个线程
thread1.start();
thread2.start();
thread3.start();
latch.await(); // 当前线程等待,直到计数器变为零
System.out.println("All workers have completed their tasks.");
}
}
class WorkerThread extends Thread {
private final CountDownLatch latch;
public WorkerThread(CountDownLatch latch) {
this.latch = latch;
}
@Override
public void run() {
try {
// 模拟任务执行
Thread.sleep(1000);
System.out.println("Task completed by " + Thread.currentThread().getName());
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
latch.countDown(); // 计数器减一
}
}
}
```
在上面的示例中,创建了一个计数器初始值为3的 `CountDownLatch` 对象,并创建了三个工作线程。每个线程执行任务后,通过 `countDown()` 方法将计数器减一。最后,在主线程中调用 `await()` 方法使主线程等待,直到计数器变为零后继续执行。输出结果类似于:
```
Task completed by Thread-0
Task completed by Thread-1
Task completed by Thread-2
All workers have completed their tasks.
```
这样就实现了主线程等待所有工作线程完成任务的效果。
阅读全文
相关推荐
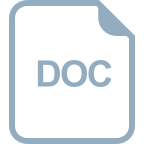
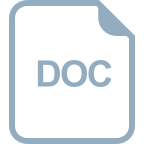
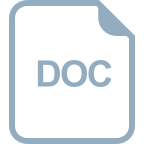






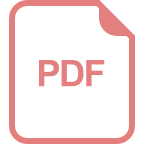
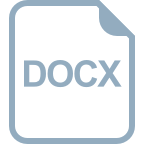
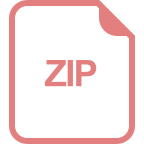
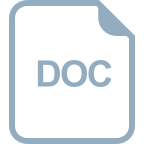





