用Python语言写一个完全背包算法,不导入库就可以使用,并且输出所放物品和价值
时间: 2024-10-13 15:15:09 浏览: 11
在Python中,我们可以手动编写一个基本的完全背包算法,不依赖于内置库。这里我们创建一个简单的函数`knapsack()`来实现这个过程。完全背包是指每个物品可以取任意次数,而不仅仅是取一次。
```python
def knapsack(capacity, weights, values, n):
# 创建二维数组,用于存储动态规划的结果
dp = [[0 for _ in range(capacity + 1)] for _ in range(n + 1)]
# 动态规划遍历所有物品和背包容量
for i in range(1, n + 1):
for w in range(1, capacity + 1):
if weights[i - 1] <= w:
# 如果当前物品重量小于等于剩余背包容量,则可以选择放入,取最大价值
dp[i][w] = max(dp[i - 1][w], dp[i - 1][w - weights[i - 1]] + values[i - 1])
else:
# 否则,如果不选择该物品,价值不变
dp[i][w] = dp[i - 1][w]
# 返回最优解
return dp[n][capacity], get_items(dp, capacity, weights, values)
def get_items(dp, capacity, weights, values, current_weight=0, item_index=-1 or current_weight > capacity:
return []
elif dp[item_index][current_weight] == dp[item_index - 1][current_weight]:
return get_items(dp, capacity, weights, values, current_weight, item_index - 1)
else:
result = [item_index]
return get_items(dp, capacity, weights, values, current_weight - weights[item_index], item_index + 1) + result
# 使用示例
capacity = 50
weights = [10, 20, 30]
values = [60, 100, 120]
n = len(weights)
optimal_value, selected_items = knapsack(capacity, weights, values, n)
print(f"最优价值: {optimal_value}")
print("选出的物品及价值:")
for i in selected_items:
print(f"物品{i}: {weights[i]}, 价值{values[i]}")
阅读全文
相关推荐
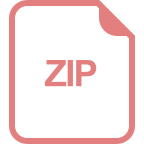
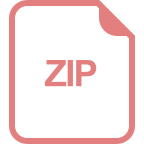
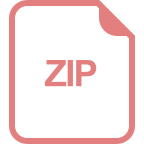
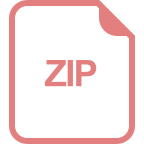
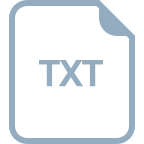
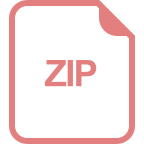
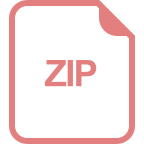
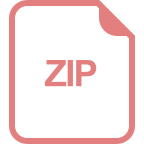
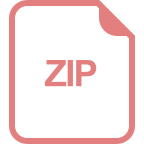
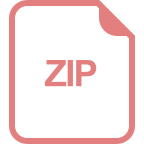
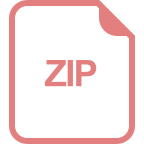
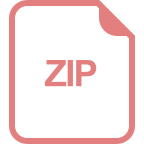
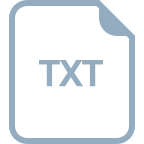
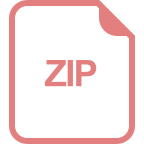
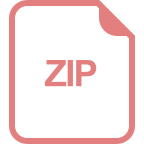