C# WPF检查对已经添加好数据的DataGrid中字段CapComponentID是否存在重复数据 如存在则报错
时间: 2024-09-27 14:05:34 浏览: 35
在C# WPF中,如果你想要在DataGrid中检查新添加的数据项是否违反了CapComponentID不允许有重复规则,你可以使用`ICollectionView`或者`ICollectionViewSource`的特性配合`INotifyDataErrorInfo`接口。以下是一种基本的步骤:
1. 首先,确保你的`DataGrid`绑定到一个`ICollectionView`或者自定义的`ICollectionViewSource`实例上。
2. 给你的数据实体(例如`CapComponent`类)添加`INotifyDataErrorInfo`接口的实现。这通常意味着你需要为`CapComponentID`属性添加验证规则,并实现`GetErrors()`和`HasErrors`方法。
```csharp
public class CapComponent : INotifyDataErrorInfo
{
public int CapComponentID { get; set; }
// 添加其他属性...
private List<string> _validationErrors;
public string this[string columnName]
{
get
{
if (columnName == "CapComponentID")
{
if (!IsCapComponentIDUnique())
return "CapComponentID已存在";
else
return null;
}
return null;
}
}
public bool HasErrors => _validationErrors?.Any() ?? false;
public IEnumerable<string> GetErrors(string propertyName)
{
if (propertyName == "CapComponentID" && IsCapComponentIDUnique())
yield break;
foreach (string error in _validationErrors)
{
yield return error;
}
}
private bool IsCapComponentIDUnique()
{
// 这里可以查询数据库或集合中是否存在相同的CapComponentID,如果有返回false,否则返回true
// 示例:
// var exists = YourDatabaseContext.CapComponents.Any(c => c.CapComponentID == CapComponentID);
// return !exists;
}
}
```
3. 在需要处理用户输入的地方,比如`TextBox`的`LostFocus`事件或者`Button`的点击事件中,检查`INotifyDataErrorInfo`是否有错误并显示给用户。
```csharp
private void textBoxCapComponentID_LostFocus(object sender, RoutedEventArgs e)
{
if (((TextBox)sender).Text.IsNullOrWhitespace())
{
// 处理空值错误...
}
else
{
if (((CapComponent)objDataContext).HasErrors)
{
MessageBox.Show(((CapComponent)objDataContext)["CapComponentID"]);
}
}
}
private void saveButton_Click(object sender, RoutedEventArgs e)
{
if (!((CapComponent)objDataContext).HasErrors)
{
// 如果没有错误,保存数据...
}
else
{
// 显示错误信息...
}
}
```
阅读全文
相关推荐
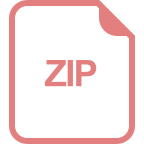
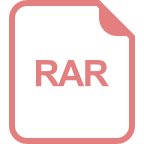
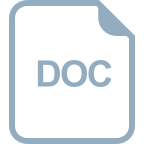
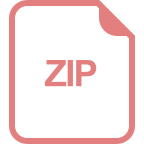
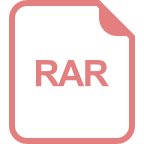
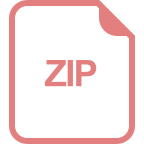
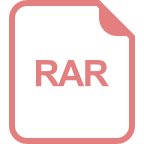
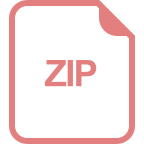
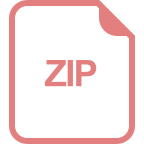
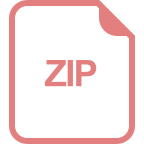
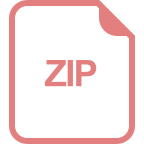
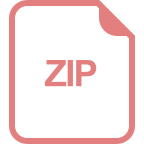
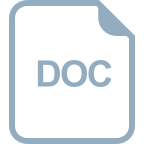
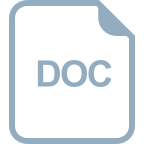



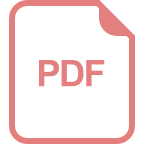
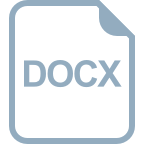