excel表格python生成词云
时间: 2024-12-23 15:12:48 浏览: 2
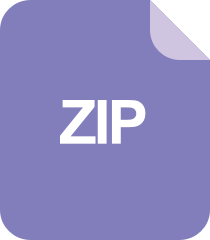
python:从excel中提取高频词生成词云
在Excel表格中创建词云通常需要借助Python的数据处理库pandas以及可视化库matplotlib和wordcloud。以下是基本步骤:
1. **数据准备**:
- 首先,你需要将Excel表格中的文本数据读取到Python环境中。可以使用pandas库的`read_excel`函数。
```python
import pandas as pd
df = pd.read_excel('your_excel_file.xlsx', sheet_name='Sheet1')
text_column = df['Your Text Column'] # 假设你的文本数据在名为'Your Text Column'的列
```
2. **数据预处理**:
- 清洗文本数据,去除标点符号、数字和特殊字符,并转为小写。
```python
import re
text = ' '.join([re.sub(r'\W+', '', str(cell)).lower() for cell in text_column])
```
3. **生成词频**:
- 使用`collections.Counter`计算每个单词的频率。
```python
from collections import Counter
word_counts = Counter(text.split())
```
4. **创建词云**:
- 使用wordcloud库创建词云图。
```python
from wordcloud import WordCloud
wordcloud = WordCloud(width=800, height=600, background_color='white').generate_from_frequencies(word_counts)
```
5. **保存和显示词云**:
- 可以选择保存为图片文件或直接在matplotlib上显示。
```python
import matplotlib.pyplot as plt
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis("off")
plt.savefig('wordcloud.png')
# 或者直接显示
plt.show()
```
阅读全文
相关推荐
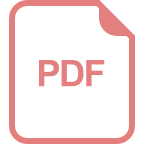
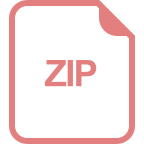





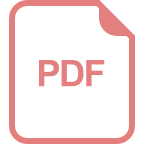
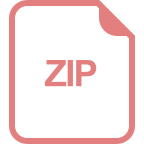
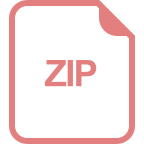
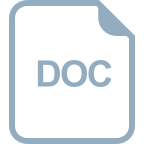
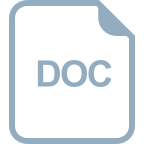





