springboot文件上传ftp
时间: 2023-08-09 20:12:42 浏览: 114
回答: 在Spring Boot中实现文件上传到FTP服务器的方法有多种。其中一种方法是使用commons-net库来实现FTP上传功能。首先,你需要在你的项目中添加commons-net的依赖。[1]然后,你可以创建一个FTPClient对象,并使用connect()方法连接到FTP服务器,使用login()方法进行登录。接下来,你可以使用changeWorkingDirectory()方法改变工作目录,使用setFileType()方法设置文件类型为二进制文件,使用enterLocalPassiveMode()方法开启被动模式。最后,使用storeFile()方法上传文件到FTP服务器。[1]另一种方法是将MultipartFile转化为File对象,然后调用ftpUtil.uploadToFtp()方法上传文件到FTP服务器。[2]在这个方法中,你需要提供FTP服务器的路径、新文件名和文件对象。[3]这样就可以实现Spring Boot文件上传到FTP服务器的功能。
相关问题
springboot中使用FTP上传文件
在Spring Boot应用中使用FTP上传文件可以使用Apache Commons Net库提供的FTP客户端实现。以下是一个简单示例:
1. 添加依赖
在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>3.6</version>
</dependency>
```
2. 创建FTP客户端
使用以下代码创建FTP客户端:
```java
public class FtpClientHelper {
private String server;
private int port;
private String username;
private String password;
private FTPClient ftpClient;
public FtpClientHelper(String server, int port, String username, String password) {
this.server = server;
this.port = port;
this.username = username;
this.password = password;
ftpClient = new FTPClient();
}
public void connect() throws IOException {
ftpClient.connect(server, port);
ftpClient.login(username, password);
}
public void disconnect() throws IOException {
ftpClient.logout();
ftpClient.disconnect();
}
public boolean isConnected() {
return ftpClient.isConnected();
}
public void uploadFile(File file, String remoteDir) throws IOException {
InputStream inputStream = new FileInputStream(file);
ftpClient.storeFile(remoteDir + "/" + file.getName(), inputStream);
inputStream.close();
}
}
```
3. 使用FTP客户端上传文件
在需要上传文件的地方,创建FTP客户端实例并连接到FTP服务器:
```java
FtpClientHelper ftpClientHelper = new FtpClientHelper("ftp.example.com", 21, "username", "password");
ftpClientHelper.connect();
```
然后,使用FTP客户端上传文件:
```java
File file = new File("path/to/local/file");
String remoteDir = "/path/to/remote/dir";
ftpClientHelper.uploadFile(file, remoteDir);
```
最后,记得在上传完成后断开FTP连接:
```java
ftpClientHelper.disconnect();
```
springboot上传文件到ftp
Spring Boot可以使用Apache Commons Net库来上传文件到FTP服务器。以下是一个简单的示例:
1. 添加依赖
在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>3.6</version>
</dependency>
```
2. 编写上传代码
在Spring Boot应用程序中,您可以使用以下代码将文件上传到FTP服务器:
```
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.io.InputStream;
@Service
public class FtpService {
private static final String FTP_HOST = "ftp.example.com";
private static final String FTP_USERNAME = "username";
private static final String FTP_PASSWORD = "password";
public void uploadFile(MultipartFile file) throws IOException {
FTPClient ftpClient = new FTPClient();
try {
ftpClient.connect(FTP_HOST);
ftpClient.login(FTP_USERNAME, FTP_PASSWORD);
ftpClient.enterLocalPassiveMode();
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
String fileName = file.getOriginalFilename();
InputStream inputStream = file.getInputStream();
ftpClient.storeFile(fileName, inputStream);
} finally {
ftpClient.logout();
ftpClient.disconnect();
}
}
}
```
在上面的代码中,我们使用FTPClient类连接到FTP服务器,然后使用storeFile()方法将文件上传到服务器。
3. 测试上传
您可以使用以下代码测试上传:
```
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
@RestController
public class FileUploadController {
@Autowired
private FtpService ftpService;
@PostMapping("/upload")
public String uploadFile(@RequestParam("file") MultipartFile file) throws IOException {
ftpService.uploadFile(file);
return "File uploaded successfully";
}
}
```
在上面的代码中,我们使用Spring MVC的@RequestParam注释将上传的文件作为参数传递给uploadFile()方法。
希望这可以帮助您将文件上传到FTP服务器。
阅读全文
相关推荐
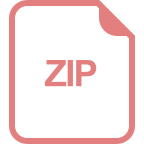
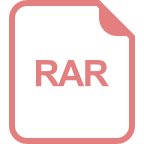
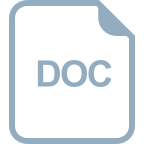


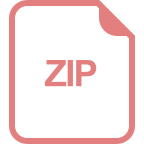
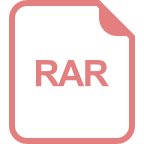
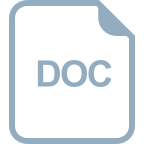
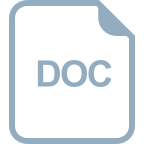
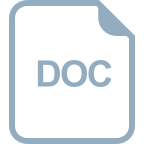
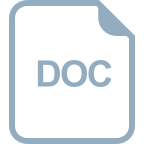
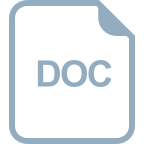

