pyqt5如何设计用一个图像超分辨率训练好的模型,应用到PYQT5设计的页面实现交互,随便输入一张图像,经过pqt5所调用训练好的图像超分辨率图像模型pth,生成一个超分辨率图像并展示在pyqt5的展示框
时间: 2024-10-12 19:05:46 浏览: 28
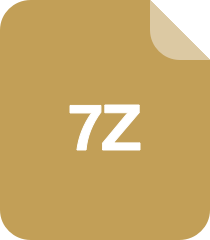
QT好看的背景图片,PYQT界面设计
在PyQt5中将一个预训练的图像超分辨率模型集成到应用程序中,你需要按照以下步骤操作:
1. **安装必要的库**:
首先,确保已安装了`torch`, `torchvision`, 和 PyQt5。你可以使用pip来安装:
```
pip install torch torchvision opencv-python pyqt5
```
2. **加载模型**:
导入所需模块,并加载预训练的超分辨率模型。假设模型保存为.pth文件,可以这样加载:
```python
import torch
model = torch.load('your_model.pth', map_location=torch.device('cpu')) if not torch.cuda.is_available() else torch.load('your_model.pth')
```
3. **图像处理函数**:
创建一个函数,该函数接收用户输入的图片,通过模型进行增强处理,然后转换回PyQt5能显示的格式:
```python
from PIL import Image
import cv2
def upscale_image(image_path, model):
img = cv2.imread(image_path)
# 将OpenCV图像转为Tensor
img_tensor = torch.from_numpy(img).float()
# 执行模型预测
upscaled_img = model(img_tensor.unsqueeze(0))
# 可能需要对结果做一些调整(例如从CHW到HWC)
upscaled_img = np.transpose(upscaled_img.squeeze().numpy(), (1, 2, 0))
return upscaled_img
def show_in_qt(upscaled_img):
# 将图片转为QImage对象
scaled_img = QImage(upscaled_img.data, upscaled_img.shape[1], upscaled_img.shape[0], QImage.Format_RGB888)
pixmap = QPixmap(scaled_img)
widget = QLabel()
widget.setPixmap(pixmap)
return widget
```
4. **PyQt5界面集成**:
在PyQt5窗口上添加一个按钮或选择文件输入控件,当用户点击或选择文件后,调用上述函数并将结果显示出来:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QVBoxLayout, QLabel
class YourApp(QWidget):
def __init__(self):
super().__init__()
self.init_ui()
def init_ui(self):
layout = QVBoxLayout()
self.input_button = QPushButton("上传图像")
self.input_button.clicked.connect(self.upload_image)
layout.addWidget(self.input_button)
self.result_label = QLabel()
layout.addWidget(self.result_label)
self.setLayout(layout)
def upload_image(self):
file_dialog = QFileDialog()
if file_dialog.exec():
selected_file = file_dialog.selectedFiles()[0]
upsampled_img = upscale_image(selected_file, model)
self.result_label.setPixmap(show_in_qt(upsampled_img))
app = QApplication(sys.argv)
window = YourApp()
window.show()
sys.exit(app.exec_())
```
阅读全文
相关推荐
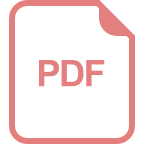
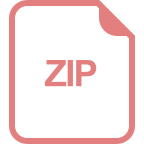

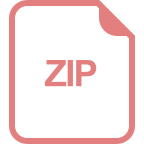
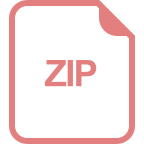
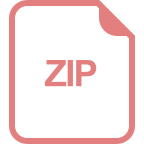
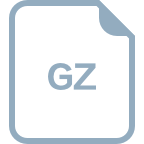
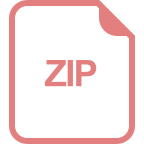
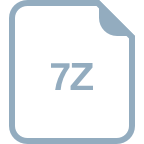
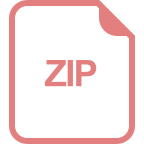
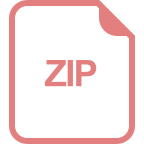
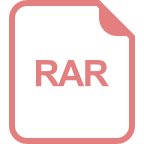
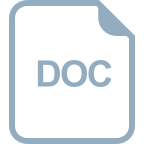
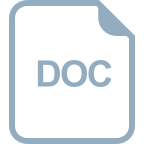
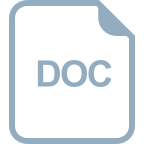
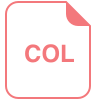
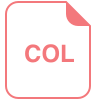
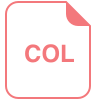