fastapi中response_model
时间: 2024-04-24 15:20:35 浏览: 14
在FastAPI中,response_model是一个用于指定API响应模型的装饰器参数。它允许你定义一个Pydantic模型,用于描述API的响应数据结构。当你使用response_model装饰器参数时,FastAPI会自动将响应数据转换为指定的模型类型,并进行验证。
使用response_model的好处是,它可以帮助你在编写API时更好地定义和验证返回的数据结构,提高代码的可读性和可维护性。此外,它还可以自动生成API文档,并提供了一些便捷的功能,如自动转换数据类型和验证数据的有效性。
下面是一个使用response_model的示例:
```python
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class Item(BaseModel):
name: str
price: float
@app.post("/items/", response_model=Item)
async def create_item(item: Item):
# 处理创建item的逻辑
return item
```
在上面的示例中,我们定义了一个名为Item的Pydantic模型,用于描述API的响应数据结构。在create_item函数上使用response_model装饰器参数,指定了API的响应模型为Item。当请求成功时,FastAPI会自动将返回的数据转换为Item类型,并进行验证。
相关问题
fastapi response_model Generic自定义返回JSON数组
如果你想要自定义 FastAPI 的 response_model 参数来返回一个 JSON 数组,你可以使用 List[Response[Dict[str, Any]]] 来实现。这里的 Dict[str, Any] 表示返回的 JSON 对象可以包含任意的键值对,具体的键和值可以根据你的需要进行定义,示例代码如下:
```python
from typing import Generic, List, TypeVar, Dict, Any
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
T = TypeVar('T')
class Response(Generic[T]):
data: T
code: int = 0
msg: str = "success"
class User(BaseModel):
name: str
age: int
@app.get("/users/", response_model=List[Response[Dict[str, Any]]])
async def read_users():
users = [{"name": "Alice", "age": 20}, {"name": "Bob", "age": 25}]
response = [Response[Dict[str, Any]](data=user_dict) for user_dict in users]
return response
```
在这个示例代码中,我们使用了一个类似于之前的 Response 泛型类,但是 data 属性的类型是 Dict[str, Any],这样就可以包含任意的键值对。在 read_users 函数中,我们使用了 List[Response[Dict[str, Any]]] 作为 response_model 参数,表示返回一个 Response 泛型类的列表,每个 Response 对象的 data 属性都是一个包含任意键值对的字典。我们根据实际数据构造了一个 Response 泛型类的列表,并将其作为返回值。
使用这种方式可以方便地自定义返回的 JSON 数组,可以根据实际需要定义不同的 Response 类型,以及每个 Response 对象的 data 属性的键值对。
fastapi response_model typing泛型实现统一json格式,类似Java的Result规定统一返回
你可以使用FastAPI中的Response类来实现统一的JSON格式返回。Response类允许你定义返回的数据类型以及HTTP状态码,这样你就可以在整个应用程序中使用统一的返回格式。
下面是一个示例,展示如何使用Response类和自定义的Result类来实现统一的JSON格式返回:
```python
from fastapi import FastAPI, Response
from pydantic import BaseModel
from typing import Generic, TypeVar, List
app = FastAPI()
T = TypeVar('T')
class Result(Generic[T]):
success: bool
data: T
error: str = None
class Item(BaseModel):
name: str
description: str = None
@app.post("/items/", response_model=Result[List[Item]])
async def create_item(item: Item, response: Response):
items = [Item(name=item.name, description=item.description), Item(name=item.name, description=item.description)]
result = Result(success=True, data=items)
response.status_code = 200 if result.success else 400
return result
```
在上面的代码中,我们定义了一个自定义的Result类,用于统一的JSON格式返回。在create_item路由函数中,我们将response_model参数设置为Result[List[Item]],这意味着返回的数据是一个Result对象,其中data属性是一个Item对象的列表。在函数中,我们创建了一个包含两个Item对象的列表,并将其赋值给Result对象的data属性。然后,我们将Result对象作为返回值返回,并将HTTP状态码也设置为200或400,具体取决于Result对象的success属性。
这样,你就可以在整个应用程序中使用统一的JSON格式返回了。
相关推荐
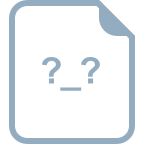
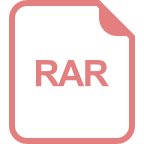
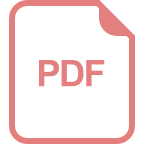












