vue3 store模块化
时间: 2023-08-18 11:14:47 浏览: 46
Vue 3中的Store模块化是通过使用新的Composition API来实现的。在Vue 2中,我们通常使用Vuex来管理应用程序的状态,但在Vue 3中,我们可以使用Composition API来更好地组织和管理状态。
首先,我们需要创建一个新的store模块。可以在项目的根目录下创建一个名为`store`的文件夹,并在其中创建一个名为`index.js`的文件。
在`index.js`中,我们可以使用`createStore`函数来创建一个新的store实例,并导出它。例如:
```javascript
import { createStore } from 'vuex';
const store = createStore({
// state, mutations, actions, getters, 等等
});
export default store;
```
然后,我们可以在应用程序的入口文件(通常是`main.js`)中导入并使用这个store实例。例如:
```javascript
import { createApp } from 'vue';
import store from './store';
const app = createApp(App);
app.use(store);
app.mount('#app');
```
现在,我们可以在store模块中定义我们的状态、mutations、actions等。例如:
```javascript
import { reactive } from 'vue';
export default {
state: reactive({
count: 0,
}),
mutations: {
increment(state) {
state.count++;
},
decrement(state) {
state.count--;
},
},
actions: {
asyncIncrement(context) {
setTimeout(() => {
context.commit('increment');
}, 1000);
},
},
};
```
这只是一个简单的示例,你可以根据你的应用程序需求来定义更多的模块和状态。
最后,我们可以在组件中使用`useStore`函数来访问和操作store中的状态。例如:
```javascript
import { useStore } from 'vuex';
export default {
setup() {
const store = useStore();
// 获取状态
const count = computed(() => store.state.count);
// 调用mutation
const increment = () => store.commit('increment');
// 调用action
const asyncIncrement = () => store.dispatch('asyncIncrement');
return {
count,
increment,
asyncIncrement,
};
},
};
```
这就是Vue 3中使用Composition API实现的Store模块化的基本步骤。希望对你有所帮助!如有疑问,请随时提问。
相关推荐
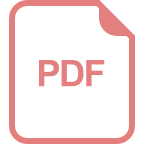
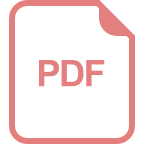
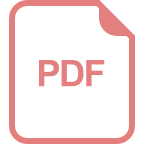














