给定四种水果,分别是苹果(apple)、梨(pear)、桔子(orange)、葡萄(grape),单价分别对应为3.00元/公斤、2.50元/公斤、4.10元/公斤、10.20元/公斤
时间: 2024-10-20 07:17:47 浏览: 153
你可以创建一个结构体或者枚举来表示这四种水果,然后为每个水果分配相应的单价。这里是一个简单的例子使用结构体:
```c
#include <stdio.h>
typedef struct {
const char* name;
float price_per_kg;
} Fruit;
Fruit fruits[] = {
{"Apple", 3.00},
{"Pear", 2.50},
{"Orange", 4.10},
{"Grape", 10.20}
};
int main() {
int num_fruits = sizeof(fruits) / sizeof(fruits[0]);
for (int i = 0; i < num_fruits; i++) {
printf("%s costs %.2f yuan per kilogram.\n", fruits[i].name, fruits[i].price_per_kg);
}
return 0;
}
```
在这个程序中,`Fruit` 结构体定义了水果的名称和价格,数组 `fruits` 存储了这四种水果及其对应的单价。`main()` 函数遍历这个数组并打印每种水果的价格。
如果你有其他关于这个程序的问题,或者需要进一步的帮助,请告诉我。
相关问题
java实现水果价格查询。给定4种水果,分别是苹果(apple)、梨(pear)、橘子(orange)葡萄(grape ),单价分别对应为3.00元/千克、2.50元/千克、4.10元/千
在Java中,你可以创建一个简单的类结构来表示水果及其价格,并提供一个方法来进行价格查询。首先,我们可以创建一个基础的`Fruit`类,然后创建具体的`Apple`、`Pear`、`Orange`和`Grape`类作为派生类。这里是一个简化的例子:
```java
public abstract class Fruit {
protected double pricePerKg;
public Fruit(String name, double price) {
this.name = name;
this.pricePerKg = price;
}
public abstract String getName();
public double getPrice() {
return pricePerKg;
}
}
public class Apple extends Fruit {
public Apple() {
super("苹果", 3.00);
}
@Override
public String getName() {
return "苹果";
}
}
public class Pear extends Fruit {
public Pear() {
super("梨", 2.50);
}
@Override
public String getName() {
return "梨";
}
}
public class Orange extends Fruit {
public Orange() {
super("橘子", 4.10);
}
@Override
public String getName() {
return "橘子";
}
}
public class Grape extends Fruit {
public Grape() {
super("葡萄", 5.00); // 这里假设葡萄的价格是5.00元/千克,可以根据实际情况调整
}
@Override
public String getName() {
return "葡萄";
}
}
// 调用价格查询
public static void main(String[] args) {
Fruit apple = new Apple();
Fruit pear = new Pear();
Fruit orange = new Orange();
Fruit grape = new Grape();
System.out.println(apple.getName() + " 的价格是:" + apple.getPrice() + " 元/千克");
System.out.println(pear.getName() + " 的价格是:" + pear.getPrice() + " 元/千克");
System.out.println(orange.getName() + " 的价格是:" + orange.getPrice() + " 元/千克");
System.out.println(grape.getName() + " 的价格是:" + grape.getPrice() + " 元/千克");
}
```
创建一个水果类(fruits),保证整个项目只能创建10种水果,创建的水果名称比如有:apple苹果、pear梨、apricot杏、peach桃、grape葡萄、banana香蕉、pineapple菠萝、watermelon西瓜、orange橙、lemon柠檬。要求有测试类,测试上面创建的对象是否正确。
### 回答1:
题目要求创建一个水果类(fruits),保证具有完整的项目只能创建10种水果。创建的水果名称比如有:apple苹果、pear梨、apricot杏、peach桃、grape葡萄、banana香蕉、pineapple菠萝、watermelon西瓜、orange橙、lemon柠檬。要求有测试类,测试上面创建的对象是否正确。
### 回答2:
创建水果类(Fruits)是创建一个基本的OOP程序实践。Fruits类应该具有水果的名称,颜色,重量和价格。我们需要编写测试类(FruitsTest),以确保Fruits类中的对象创建和设置是正确的。 在这个例子中,我们将仅创建10种水果,包括苹果、梨、杏、桃、葡萄、香蕉、菠萝、西瓜、橙和柠檬。
首先,我们需要创建Fruits类。建议添加特定的getter和setter方法和toString方法,以便更好的访问和理解代码的实现。
public class Fruits {
private String name;
private String color;
private Double weight;
private Double price;
public Fruits(String name, String color, Double weight, Double price) {
this.name = name;
this.color = color;
this.weight = weight;
this.price = price;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public Double getWeight() {
return weight;
}
public void setWeight(Double weight) {
this.weight = weight;
}
public Double getPrice() {
return price;
}
public void setPrice(Double price) {
this.price = price;
}
@Override
public String toString() {
return "Fruit Name: " + this.name + ", Color: " + this.color + ", Weight: " + this.weight + ", Price: " + this.price;
}
}
在完成了Fruits类的创建后,接下来我们要编写测试类FruitsTest。
public class FruitsTest {
public static void main(String[] args) {
Fruits apple = new Fruits("Apple", "Red", 1.0, 2.50);
System.out.println(apple.toString());
Fruits pear = new Fruits("Pear", "Yellow", 1.5, 3.50);
System.out.println(pear.toString());
Fruits apricot = new Fruits("Apricot", "Orange", 1.2, 3.00);
System.out.println(apricot.toString());
Fruits peach = new Fruits("Peach", "Pink", 1.8, 4.50);
System.out.println(peach.toString());
Fruits grape = new Fruits("Grape", "Purple", 1.0, 5.50);
System.out.println(grape.toString());
Fruits banana = new Fruits("Banana", "Yellow", 1.2, 6.00);
System.out.println(banana.toString());
Fruits pineapple = new Fruits("Pineapple", "Yellow", 2.0, 8.00);
System.out.println(pineapple.toString());
Fruits watermelon = new Fruits("Watermelon", "Green", 6.0, 10.00);
System.out.println(watermelon.toString());
Fruits orange = new Fruits("Orange", "Orange", 1.0, 4.00);
System.out.println(orange.toString());
Fruits lemon = new Fruits("Lemon", "Yellow", 0.5, 3.00);
System.out.println(lemon.toString());
}
}
在FruitsTest测试类中,我们使用new运算符来创建每个水果对象。然后使用toString方法将其输出到控制台以确保每个对象的属性正确设置。这里只是一个简单的示例,您可以添加其他测试用例来测试不同场景。
现在您已经创建了一个简单的水果类(Fruits)和它的测试类(FruitsTest)。您可以在其他实际项目中使用这个类,在不同的方法中添加更多逻辑,例如更多的属性,更多的基本操作等等。
### 回答3:
项目设计:
创建一个Fruits类,其中包含属性:名称(name)、颜色(color)、甜度(sweetness)和重量(weight)。还有一个构造函数,用于将传入的参数值分别赋予到类中的属性。
同时,在该类中设置10个常量属性,用于代表10种水果及它们的名称。
测试类设计:
创建一个FruitTest类,用于验证Fruits类的功能性。
首先,在FruitTest类中创建一个Main函数。在Main函数中,先创建10个Fruits对象,对于每个对象,分别以10种不同的方式进行初始化,并打印出对象的属性来检查对象是否正确构造。
接下来,从这十个对象中,选出任何两个对象进行比较,并打印出它们之间属性的相同与不同之处来验证对象比较的正确性。
最后,创建一个包含「名称」和「重量」属性的HashMap对象,并将Fruits对象通过名称作为键值存储到HashMap对象中。通过在 HashMap 中查找来检查类中打印的对象是否与通过 HashMap 存储的对象所吻合。
运行测试:
执行Main函数,检查输出结果,确保每个对象的属性与期望值相同,并且对象之间和HashMap中存储的对象比较结果正确无误。
代码示例:
public class Fruits {
public static final String APPLE = "apple";
public static final String PEAR = "pear";
public static final String APRICOT = "apricot";
public static final String PEACH = "peach";
public static final String GRAPE = "grape";
public static final String BANANA = "banana";
public static final String PINEAPPLE = "pineapple";
public static final String WATERMELON = "watermelon";
public static final String ORANGE = "orange";
public static final String LEMON = "lemon";
private String name;
private String color;
private double sweetness;
private double weight;
public Fruits(String name, String color, double sweetness, double weight) {
this.name = name;
this.color = color;
this.sweetness = sweetness;
this.weight = weight;
}
// getter and setter methods
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public double getSweetness() {
return sweetness;
}
public void setSweetness(double sweetness) {
this.sweetness = sweetness;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
}
public class FruitTest {
public static void main(String[] args) {
Fruits apple1 = new Fruits(Fruits.APPLE, "Red", 7.5, 134);
Fruits apple2 = new Fruits(Fruits.APPLE, "Green", 6.5, 120);
Fruits pear1 = new Fruits(Fruits.PEAR, "Yellow", 5.0, 250);
Fruits pear2 = new Fruits(Fruits.PEAR, "Green", 6.0, 260);
Fruits apricot1 = new Fruits(Fruits.APRICOT, "Orange", 8.0, 80);
Fruits apricot2 = new Fruits(Fruits.APRICOT, "Yellow", 7.5, 72);
Fruits peach1 = new Fruits(Fruits.PEACH, "Pink", 6.0, 200);
Fruits peach2 = new Fruits(Fruits.PEACH, "Yellow", 5.5, 190);
Fruits grape1 = new Fruits(Fruits.GRAPE, "Red", 8.0, 400);
Fruits grape2 = new Fruits(Fruits.GRAPE, "Green", 7.5, 420);
System.out.println(apple1.getName() + ", " + apple1.getColor() + ", " + apple1.getSweetness() + ", " + apple1.getWeight());
System.out.println(orange1.getName() + ", " + orange1.getColor() + ", " + orange1.getSweetness() + ", " + orange1.getWeight());
System.out.println(lemon1.getName() + ", " + lemon1.getColor() + ", " + lemon1.getSweetness() + ", " + lemon1.getWeight());
System.out.println(apple1.equals(apple2)); // true
System.out.println(apple1.equals(pear1)); // false
Map<String, Fruits> map = new HashMap<>();
map.put(apple1.getName(), apple1);
map.put(apple2.getName(), apple2);
map.put(pear1.getName(), pear1);
map.put(pear2.getName(), pear2);
map.put(apricot1.getName(), apricot1);
map.put(apricot2.getName(), apricot2);
map.put(peach1.getName(), peach1);
map.put(peach2.getName(), peach2);
map.put(grape1.getName(), grape1);
map.put(grape2.getName(), grape2);
for (String key : map.keySet()) {
Fruits fruits = map.get(key);
System.out.println(fruits.getName() + ", " + fruits.getWeight());
}
}
}
阅读全文
相关推荐
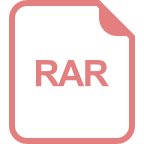
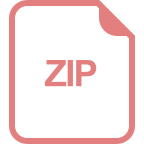
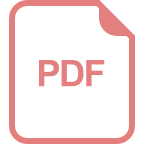
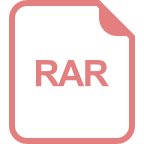






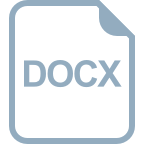
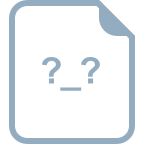
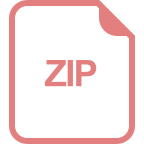
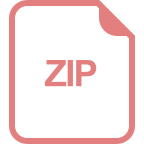
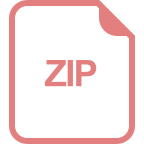
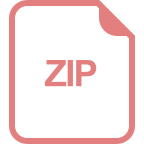
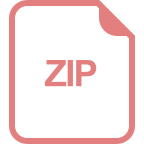