经典进程的同步问题按照实验要求完成生产者与消费者问题模型实现,并验证程序执行结果
时间: 2024-12-19 16:18:24 浏览: 21
经典生产者-消费者问题是一种经典的并发控制问题,它描述了一个系统中存在两个实体,一个是生产者,负责生成数据放入缓冲区;另一个是消费者,从缓冲区取出数据进行处理。在实现这一模型时,通常会用到线程同步机制,如互斥量(mutex)、条件变量(condition variables)等。
在Python中,你可以使用`threading`模块以及`queue`模块来模拟这个场景:
```python
import threading
from queue import Queue
class Producer(threading.Thread):
def __init__(self, queue):
super().__init__()
self.queue = queue
self.daemon = True
def run(self):
for _ in range(10): # 生产者生产10次
item = "Product" # 生产的数据
with self.queue.not_empty: # 获取生产权限
self.queue.put(item)
print(f"Producer produced {item} and put it into the queue.")
self.queue.not_full.notify() # 通知消费者有新商品
class Consumer(threading.Thread):
def __init__(self, queue):
super().__init__()
self.queue = queue
self.daemon = True
def run(self):
while True:
with self.queue.empty: # 获取消费权限
if not self.queue.empty():
item = self.queue.get()
print(f"Consumer consumed {item} from the queue.")
else:
self.queue.not_full.wait() # 等待有商品
if __name__ == "__main__":
buffer_size = 5
queue = Queue(maxsize=buffer_size)
producer = Producer(queue)
consumer = Consumer(queue)
producer.start()
consumer.start()
producer.join()
consumer.join()
print("Both threads finished.")
```
在这个例子中,我们创建了两个线程分别代表生产者和消费者。`not_empty`和`not_full`是`Condition`对象,用于控制同步。当队列为空时,消费者等待,当队列非空时,生产者才允许继续。运行这个程序可以看到生产者和消费者如何交替工作,直到生产者完成所有产品。
阅读全文
相关推荐




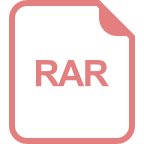

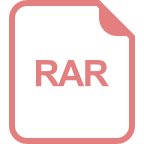
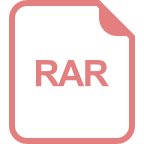









