查询当前时间船只的经纬度信息的api开发,或者使用若依 spring boot +vue2 的经纬度api开发详细代码
时间: 2024-11-09 11:30:33 浏览: 5
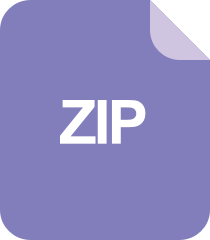
基于spring boot+vue实现小程序视频点播+直播+社区交流+评论+抢购+拍卖+短视频
要查询当前时间船只的经纬度信息,可以使用一些公开的API服务,例如Google Maps API、OpenStreetMap等。这些API通常提供实时的位置数据,包括船只的经纬度信息。
下面是一个使用Spring Boot和Vue.js开发的基本示例,展示如何调用一个假设的API来获取船只的经纬度信息。
### 后端(Spring Boot)
1. **创建Spring Boot项目**:
- 使用Spring Initializr创建一个Spring Boot项目,选择依赖项:Spring Web, Spring Boot DevTools, Lombok。
2. **添加依赖**:
在`pom.xml`中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
```
3. **创建控制器**:
创建一个控制器类来处理API请求。
```java
package com.example.shiptracking.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.example.shiptracking.service.ShipService;
@RestController
public class ShipController {
@Autowired
private ShipService shipService;
@GetMapping("/api/ship/location")
public ShipLocation getShipLocation(@RequestParam String shipId) {
return shipService.getShipLocation(shipId);
}
}
```
4. **创建服务层**:
创建一个服务类来处理业务逻辑。
```java
package com.example.shiptracking.service;
import org.springframework.stereotype.Service;
import com.example.shiptracking.model.ShipLocation;
@Service
public class ShipService {
public ShipLocation getShipLocation(String shipId) {
// 这里可以调用外部API获取船只位置信息
// 假设我们有一个方法调用外部API并返回ShipLocation对象
return callExternalApiForShipLocation(shipId);
}
private ShipLocation callExternalApiForShipLocation(String shipId) {
// 模拟调用外部API
ShipLocation location = new ShipLocation();
location.setLatitude(37.7749); // 示例纬度
location.setLongitude(-122.4194); // 示例经度
return location;
}
}
```
5. **创建模型类**:
创建一个模型类来表示船只的位置信息。
```java
package com.example.shiptracking.model;
public class ShipLocation {
private double latitude;
private double longitude;
// Getters and Setters
public double getLatitude() {
return latitude;
}
public void setLatitude(double latitude) {
this.latitude = latitude;
}
public double getLongitude() {
return longitude;
}
public void setLongitude(double longitude) {
this.longitude = longitude;
}
}
```
### 前端(Vue.js)
1. **创建Vue项目**:
使用Vue CLI创建一个Vue项目。
```bash
vue create ship-tracking-frontend
cd ship-tracking-frontend
```
2. **安装Axios**:
安装Axios用于HTTP请求。
```bash
阅读全文
相关推荐
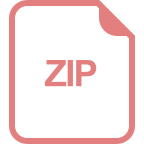
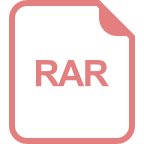

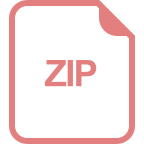
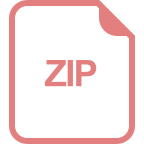
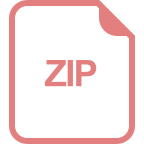
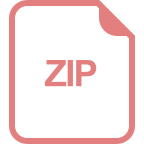
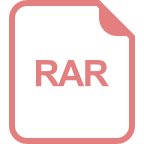
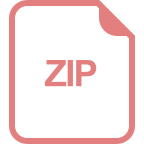
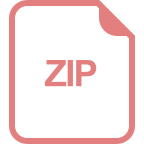
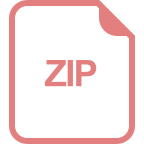
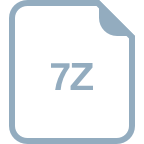
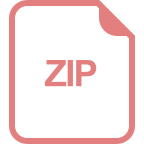
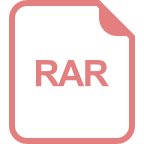