vue页面非正常关闭清除token
时间: 2024-09-23 13:06:41 浏览: 27
Vue页面的非正常关闭通常是指用户直接关闭浏览器窗口、强制刷新页面或者导航到其他页面,这种情况下,为了避免安全风险(如未经授权的持续访问),应该在适当的地方清除用户的登录凭证,比如JWT令牌(JSON Web Token)。在Vue应用中,清除token的一般做法是在组件的beforeDestroy生命周期钩子或vuex的mutation里:
```javascript
// 组件中
beforeDestroy() {
localStorage.removeItem('jwtToken'); // 如果存储在本地存储
// 或者
this.$store.dispatch('logout'); // 如果通过Vuex管理
}
// Vuex store 中的 mutation
export const logout = (state) => {
state.token = null;
localStorage.removeItem('jwtToken');
}
```
这里假设你已经将JWT token存储在了`localStorage`中,并且你在Vuex的状态管理中有相应的`logout`操作。
相关问题
vue页面缓存数据,页面关闭时清除数据
Vue页面缓存数据是指在使用Vue进行页面开发时,可以通过一些方法将页面中的数据缓存起来,以便在页面重新打开时可以快速恢复到之前的状态。而页面关闭时清除数据,则是指当用户关闭页面时,需要将缓存的数据清除,以确保下次打开页面时不会加载上次的数据。
在Vue中,可以通过使用Vuex来实现页面数据的缓存和清除。Vuex是Vue的官方状态管理库,用于在Vue应用中集中管理共享的状态。在Vuex中,可以将页面需要缓存的数据保存在state中,当页面关闭时,可以通过mutation来清除这些数据。
首先,在Vuex中定义一个用于保存页面数据的state,例如:
```javascript
state: {
cachedData: null
}
```
然后,在mutations中定义一个用于清除数据的mutation:
```javascript
mutations: {
clearData(state) {
state.cachedData = null;
}
}
```
接下来,在页面关闭时调用清除数据的mutation。可以在Vue的beforeDestroy钩子函数中调用该mutation,例如:
```javascript
beforeDestroy() {
this.$store.commit('clearData');
}
```
这样,在页面关闭时,就可以清除已经缓存的数据了。
另外需要注意,在使用Vue的路由进行页面切换时,如果需要缓存数据,可以在路由配置中设置:
```javascript
{
path: '/example',
component: Example,
meta: { keepAlive: true }
}
```
通过设置meta中的keepAlive属性为true,可以使页面在切换时保持缓存的数据。
总结起来,Vue页面缓存数据可以通过Vuex进行实现,通过将需要缓存的数据保存在state中,并在页面关闭时调用mutation来清除数据。同时,在使用Vue的路由时,还可以通过设置meta中的keepAlive属性来控制页面数据的缓存。
vue登录页面怎么获取token
在Vue登录页面中获取token的具体方式取决于你的后端API是如何设计的。一般来说,在用户完成登录验证后,后端API会返回一个包含token的响应。你可以使用Axios等工具发送POST请求,将用户的登录信息发送给后端API进行验证,然后从响应中解析出token并保存到本地存储(如localStorage)中,以便后续的API请求中使用。
以下是一个示例代码片段:
```javascript
import axios from 'axios';
// 发送登录请求
axios.post('/api/login', {
username: '用户名',
password: '密码'
}).then(response => {
// 从响应中解析出token并保存到本地存储
const token = response.data.token;
localStorage.setItem('token', token);
}).catch(error => {
console.error(error);
});
```
在后续的API请求中,你可以通过设置请求头将token发送给后端API进行验证。以下是一个示例代码片段:
```javascript
import axios from 'axios';
// 从本地存储中获取token
const token = localStorage.getItem('token');
// 设置请求头
axios.defaults.headers.common['Authorization'] = `Bearer ${token}`;
// 发送API请求
axios.get('/api/user').then(response => {
console.log(response.data);
}).catch(error => {
console.error(error);
});
```
需要注意的是,以上示例代码仅供参考,具体实现可能因后端API的设计而有所差异。
相关推荐
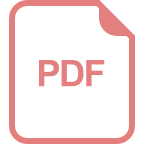
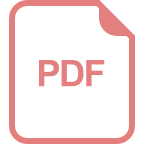
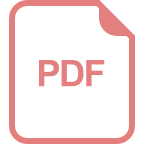












