C语言写进制矩阵计算器
时间: 2024-12-15 18:13:01 浏览: 14
C语言编写进制矩阵计算器通常会涉及到用户输入、数据处理以及矩阵操作。下面是一个简单的步骤和伪代码概述:
1. **定义函数**:
- `get_matrix_input(int base)`: 获取用户输入的矩阵,指定进制base。
- `convert_to_decimal(matrix, base)`: 将矩阵中的每个元素转换为十进制表示。
- `add_matrices(matrix1, matrix2)`: 计算两个矩阵的对应元素之和。
- `print_matrix(matrix)`: 打印矩阵。
2. **主程序流程**:
- 提示用户输入矩阵的行数和列数,以及选择的进制。
- 循环获取两个矩阵的输入,转换成十进制,并存储到二维数组中。
- 调用加法函数,对两个矩阵进行逐元素相加。
- 输出结果矩阵。
```c
#include <stdio.h>
// 定义矩阵结构体和相关的函数
typedef struct {
int rows;
int cols;
int data[ROWS][COLS];
} Matrix;
Matrix get_matrix_input(int base);
Matrix convert_to_decimal(Matrix matrix, int base);
Matrix add_matrices(Matrix matrix1, Matrix matrix2);
void print_matrix(Matrix matrix);
int main() {
// 用户输入部分
int base, rows, cols;
printf("Enter the base and dimensions for the matrices: ");
scanf("%d %d %d", &base, &rows, &cols);
// 矩阵操作
Matrix matrix1 = get_matrix_input(base);
Matrix matrix2 = get_matrix_input(base);
Matrix result = add_matrices(convert_to_decimal(matrix1, base), convert_to_decimal(matrix2, base));
// 输出结果
print_matrix(result);
return 0;
}
// ... 实现上述函数的具体代码
```
阅读全文
相关推荐
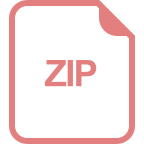
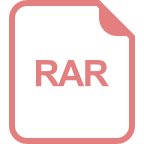
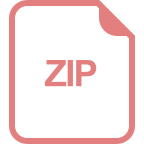
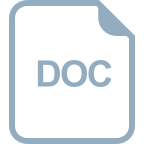

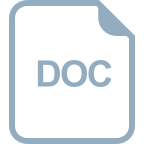
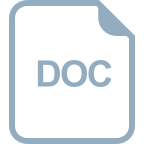
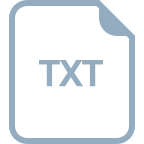
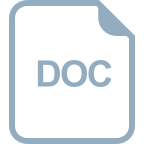
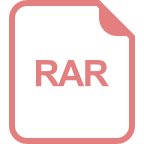
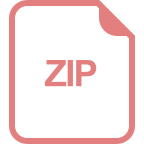
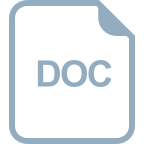
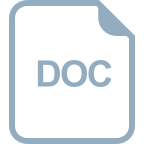
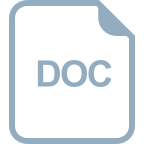
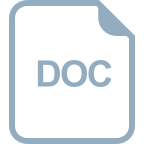
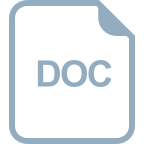
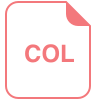
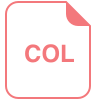
