python中如何抓取特定id的div
时间: 2024-10-06 18:00:56 浏览: 47
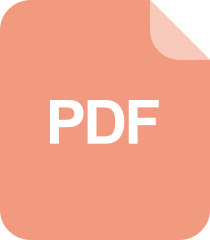
python 3利用BeautifulSoup抓取div标签的方法示例
在Python中,你可以使用BeautifulSoup库来抓取HTML页面中的特定ID的`<div>`元素。下面是一个简单的例子:
首先,你需要安装`beautifulsoup4`库,如果还没有安装可以使用pip来安装:
```bash
pip install beautifulsoup4
```
然后,你可以使用以下代码来抓取指定ID的`<div>`元素:
```python
import requests
from bs4 import BeautifulSoup
# 请求网页内容
url = "https://example.com" # 替换为你需要抓取的网页URL
response = requests.get(url)
# 使用BeautifulSoup解析HTML
soup = BeautifulSoup(response.text, 'html.parser')
# 定位到特定ID的div
target_div_id = "your_target_id" # 替换为你要找的div元素ID
target_div = soup.find('div', id=target_div_id)
# 如果找到元素,打印其文本内容
if target_div:
print(target_div.get_text())
else:
print(f"未找到ID为'{target_div_id}'的<div>元素")
# 输出结果
阅读全文
相关推荐
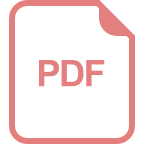
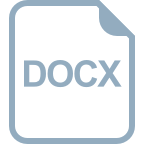
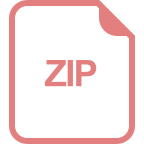
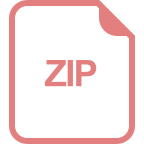
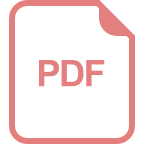
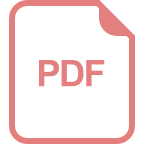
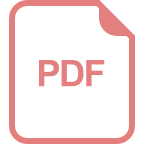
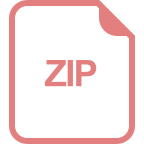
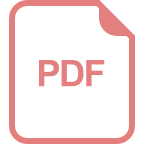
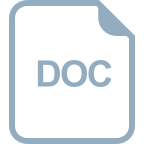
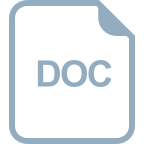
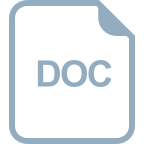
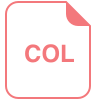
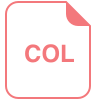



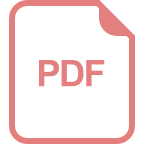
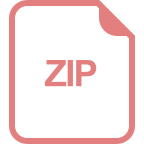