无人车路径规划算法python
时间: 2023-09-20 17:05:19 浏览: 85
无人车路径规划是指通过算法确定无人车在道路上行驶的最佳路径。在Python中,有几种常用的路径规划算法可以用于无人车路径规划。
1. A*算法:A*算法是一种启发式搜索算法,常用于无人车路径规划。它通过估计从起点到终点的代价函数来选择最佳路径。在Python中,你可以使用第三方库如networkx来实现A*算法。
2. RRT(Rapidly-exploring Random Trees)算法:RRT算法是一种基于随机采样的快速探索算法,常用于无人车路径规划。它通过扩展树结构,逐渐接近目标位置。在Python中,你可以使用第三方库如rrt库来实现RRT算法。
3. Dijkstra算法:Dijkstra算法是一种最短路径算法,可以用于无人车路径规划。它通过计算节点之间的距离来确定最佳路径。在Python中,你可以使用第三方库如networkx来实现Dijkstra算法。
4. RRT*(Rapidly-exploring Random Trees with rewire)算法:RRT*算法在RRT算法的基础上进行了改进,通过重新连接树的节点来优化路径。在Python中,你可以使用第三方库如rrt_star库来实现RRT*算法。
以上是几种常用的无人车路径规划算法,在Python中的实现可以借助相应的第三方库。具体选择哪种算法取决于你的需求和应用场景。
相关问题
无人艇路径规划的python开源代码
无人艇路径规划是指根据无人艇当前位置和目标位置,计算出最优的航行路径。Python是一门流行的编程语言,有许多开源代码库可以用于无人艇路径规划。
在Python中,有多个开源代码库可以进行路径规划,如NetworkX、Pygame和Scipy等。其中,NetworkX是一个专门用于复杂网络分析的Python库,它提供了多种用于图和网络分析的算法。而Pygame是用于开发二维游戏和图形界面的Python库,它可以用于可视化无人艇的路径规划结果。Scipy则是一个科学计算和数据分析的Python库,提供了多种数值计算和优化算法,可以用于路径规划中的数学模型。
针对无人艇路径规划,可以使用NetworkX库中的最短路径算法来计算无人艇的最优航行路径。首先,需要构建无人艇所处的环境为一个图像。可以通过定义图的节点表示水域中的位置,并在节点之间添加边表示可行航行路径。然后,调用NetworkX库的最短路径算法,传入起点和终点节点作为参数,即可得到无人艇的最优航行路径。
除了最短路径算法,还可以使用其他的路径规划算法来解决不同情况下的问题,如A*算法、蚁群算法等。这些算法可以在Scipy库中找到对应的实现代码,并结合具体问题的特点进行调用和使用。
总之,使用Python中的开源代码库,我们可以轻松实现无人艇路径规划,并根据具体要求选择不同的算法来解决不同的问题。
无人驾驶路径规划python
无人驾驶路径规划可以使用Python进行实现,以下是一些Python库和算法可以进行参考:
1. Python库:
- NumPy:用于处理数组和矩阵,可以帮助处理无人驾驶车辆的传感器数据。
- Matplotlib:可视化库,可以绘制无人驾驶车辆的路径和环境。
- OpenCV:用于图像处理和计算机视觉,可以帮助无人驾驶车辆进行环境感知和障碍物检测。
- Scikit-learn:用于机器学习和数据分析,可以帮助无人驾驶车辆进行决策制定和行为规划。
2. 路径规划算法:
- A*算法:基于启发式搜索的寻路算法,可以用于无人驾驶车辆的全局路径规划。
- RRT算法:基于随机采样的快速探索算法,可以用于无人驾驶车辆的局部路径规划。
- Dijkstra算法:基于图论的最短路径算法,可以用于无人驾驶车辆的全局路径规划。
综上所述,使用Python进行无人驾驶路径规划需要选取适合自己的算法和库,并进行合理的组合和编写代码实现。
相关推荐
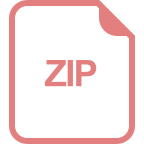
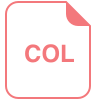
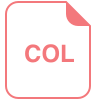
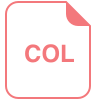
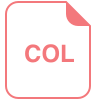
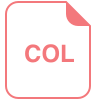







