哈希表C语言代码实现
时间: 2024-12-11 19:13:14 浏览: 44
哈希表(Hash Table),也称为散列表,是一种常见的数据结构,它通过将关键字映射到数组的特定位置来存储和查找元素。在C语言中,你可以使用数组和索引来实现简单的哈希表。以下是一个基本的单链表哈希表的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define TABLE_SIZE 10
typedef struct Node {
int key;
int value;
struct Node* next;
} HashNode;
int hashFunction(int key) {
return key % TABLE_SIZE; // 这里只是一个简单示例,实际应用中可能需要更复杂的哈希函数
}
HashNode** createHashTable() {
HashNode** table = (HashNode**)malloc(sizeof(HashNode*) * TABLE_SIZE);
for (int i = 0; i < TABLE_SIZE; i++) {
table[i] = NULL;
}
return table;
}
void insertIntoHashTable(HashNode** table, int key, int value) {
int index = hashFunction(key);
HashNode* newNode = (HashNode*)malloc(sizeof(HashNode));
newNode->key = key;
newNode->value = value;
newNode->next = table[index];
table[index] = newNode;
}
int searchHashTable(HashNode** table, int key) {
int index = hashFunction(key);
HashNode* node = table[index];
while (node != NULL) {
if (node->key == key) {
return node->value;
}
node = node->next;
}
return -1; // 如果未找到,返回-1
}
void deleteFromHashTable(HashNode** table, int key) {
int index = hashFunction(key);
HashNode* prev = NULL;
HashNode* current = table[index];
while (current != NULL && current->key != key) {
prev = current;
current = current->next;
}
if (current != NULL) {
if (prev == NULL) {
table[index] = current->next;
} else {
prev->next = current->next;
}
free(current);
}
}
// 示例用法
int main() {
HashNode** myTable = createHashTable();
insertIntoHashTable(myTable, 5, "apple");
insertIntoHashTable(myTable, 10, "banana");
int result = searchHashTable(myTable, 5); // 查找key为5的值,结果为"apple"
printf("Search result: %d\n", result);
deleteFromHashTable(myTable, 10); // 删除key为10的项
return 0;
}
```
这个例子中,我们使用了开放寻址(线性探测)解决冲突,如果哈希函数生成的位置已经有其他元素,就寻找下一个空闲位置插入。这只是一个基础版本,实际应用中可能需要处理更多复杂情况,比如链地址法(拉链法)。
阅读全文
相关推荐







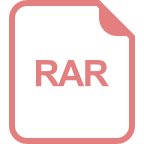

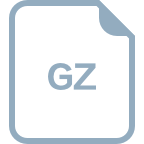
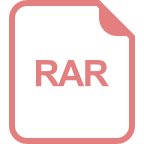





