数据结构高精度计算PI值
时间: 2024-04-29 14:16:35 浏览: 217
高精度计算PI值是一种常见的数值计算问题,可以使用多种数据结构和算法来实现。以下是一种常见的实现方式:
1. 使用无限精度整数:可以使用大整数库或自定义的数据结构来表示无限精度整数。通过使用无限精度整数,可以进行任意精度的计算,从而得到更准确的PI值。
2. 使用级数展开:PI可以通过级数展开的方式进行计算,其中最常见的是莱布尼茨级数或马青公式。这些级数可以通过循环计算来逐步逼近PI值。
3. 使用分数运算:可以使用分数运算来进行高精度计算。通过使用分数运算,可以避免浮点数运算中的舍入误差,从而得到更准确的结果。
4. 使用迭代算法:可以使用迭代算法来逐步逼近PI值。例如,可以使用蒙特卡洛方法或马青公式的迭代形式来计算PI值。
相关问题
数据结构高精度计算pi值
高精度计算pi值可以采用以下算法:
1. 利用公式pi/4 = 1 - 1/3 + 1/5 - 1/7 + ...,按照精度要求计算出前n项的和。
2. 使用二分法,求出pi的小数部分。具体做法是先将pi的整数部分计算出来,然后将pi减去整数部分,得到小数部分。然后将小数部分乘以10,得到新的小数部分,再将小数部分整除1,得到新的整数部分。重复这个过程,直到达到所需的精度。
3. 最后将整数部分和小数部分合并起来,得到pi的值。
下面是Python代码实现:
```python
def compute_pi(precision):
pi = 0
sign = 1
for i in range(precision):
term = sign / (2*i+1)
pi += term
sign = -sign
pi *= 4
return pi
def compute_pi_decimal(precision):
int_part = int(compute_pi(precision))
decimal_part = 0
for i in range(precision):
term = compute_pi(precision-i-1)
term -= int(term)
decimal_part = decimal_part*10 + int(term*10)
return int_part, decimal_part
pi = compute_pi_decimal(100)
print(pi[0], '.', pi[1])
```
这段代码可以计算出pi的前100位小数。
数据结构noj高精度计算pi值
以下是使用高精度计算方法计算pi值的代码示例:
```c++
#include <iostream>
#include <vector>
using namespace std;
const int BASE = 10000; // 每个数字占4位
const int D = 10000; // 小数点后D位
struct BigNum {
vector<int> digits; // 存储每个数字,从低位到高位
BigNum() {}
BigNum(int x) {
while (x) {
digits.push_back(x % BASE);
x /= BASE;
}
}
BigNum operator+(const BigNum& b) const {
BigNum res;
int carry = 0;
for (int i = 0; i < digits.size() || i < b.digits.size() || carry; i++) {
if (i < digits.size()) carry += digits[i];
if (i < b.digits.size()) carry += b.digits[i];
res.digits.push_back(carry % BASE);
carry /= BASE;
}
return res;
}
BigNum operator*(int x) const {
BigNum res;
int carry = 0;
for (int i = 0; i < digits.size() || carry; i++) {
if (i < digits.size()) carry += digits[i] * x;
res.digits.push_back(carry % BASE);
carry /= BASE;
}
return res;
}
BigNum operator/(int x) const {
BigNum res;
int r = 0;
for (int i = digits.size() - 1; i >= 0; i--) {
r = r * BASE + digits[i];
res.digits.push_back(r / x);
r %= x;
}
reverse(res.digits.begin(), res.digits.end());
while (res.digits.size() > 1 && res.digits.back() == 0) res.digits.pop_back();
return res;
}
friend ostream& operator<<(ostream& out, const BigNum& b) {
out << b.digits.back();
for (int i = b.digits.size() - 2; i >= 0; i--) {
out.width(4); // 每个数字占4位
out.fill('0');
out << b.digits[i];
}
return out;
}
};
int main() {
int n = (D + 3) / 4; // 需要计算的整数部分位数
BigNum a = 1, b = 1, t = 1, res = 0;
for (int i = 0; i < n; i++) {
res = res + t * a / b;
a = a * (2 * i + 1);
b = b * (i + 1);
t = t * (-1);
}
res = res * 4;
cout << res << ".";
a = a * 10;
for (int i = 0; i < D; i++) {
res = a / b;
cout.width(4);
cout.fill('0');
cout << res;
a = a % b * 10;
}
return 0;
}
```
该程序使用 BigNum 结构体存储大数,并重载了加、乘、除、输出运算符。主要思路是根据莱布尼茨级数公式计算π值的近似值,然后输出小数点后D位的数值部分。
阅读全文
相关推荐
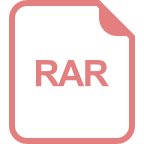
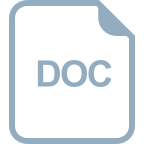
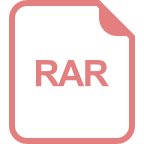
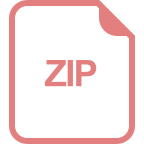
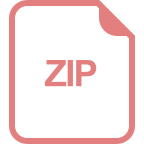
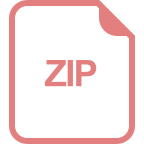
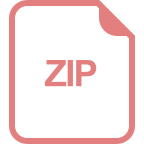
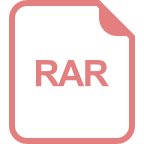
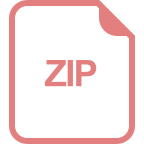
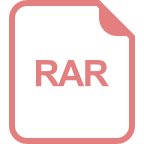
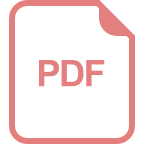
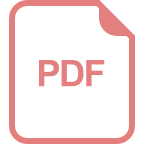
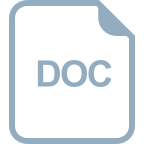
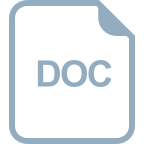
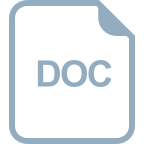