C语言利用while循环求和,计算10个随机数之和
时间: 2024-10-08 12:03:50 浏览: 34
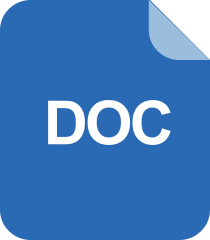
循环结构相关练习题C语言

在C语言中,你可以通过以下步骤利用`while`循环来求和10个随机数:
1. 首先,你需要包含头文件`<stdio.h>`用于输入输出以及`<stdlib.h>`用于生成随机数。
```c
#include <stdio.h>
#include <stdlib.h>
```
2. 定义一个变量来存储总和,并初始化为0。
```c
int sum = 0;
```
3. 使用`while`循环,当计数器小于10时,不断生成随机数并累加到总和上。这里可以借助`srand()`和`rand()`函数来获取随机数。
```c
srand(time(0)); // 设置随机种子,保证每次程序运行得到不同的随机数序列
while (count < 10) { // count作为循环次数的控制变量,初始值设为1
int random_num = rand() % 100; // 生成0~99之间的整数
sum += random_num;
count++; // 每次循环后,计数器加一
}
```
4. 循环结束后,打印出结果。
```c
printf("The sum of the 10 random numbers is: %d\n", sum);
```
完整的示例代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
int sum = 0, count = 1;
srand(time(0));
while (count < 10) {
int random_num = rand() % 100;
sum += random_num;
count++;
}
printf("The sum of the 10 random numbers is: %d\n", sum);
return 0;
}
```
阅读全文
相关推荐
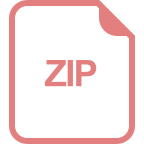
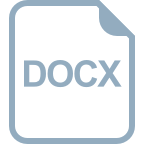

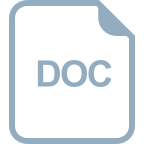
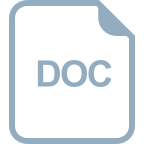
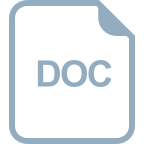
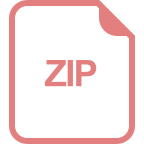
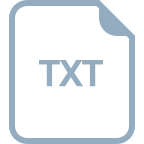
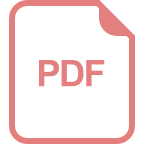
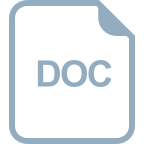
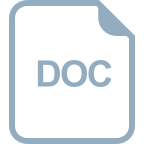
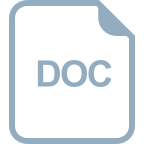
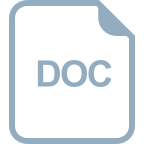
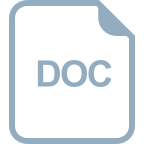
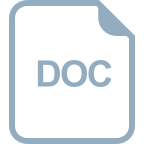
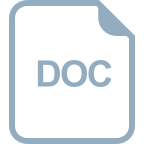
