jsqlparser-2.1.jar实现动态sql条件剔除详细例子
时间: 2024-11-27 10:09:29 浏览: 3
JSqlParser是一个强大的SQL解析库,它可以帮助你在运行时分析、修改和执行SQL语句。如果你想要通过jsqlparser实现动态SQL条件的剔除,首先你需要对SQL语句进行解析,然后筛选出不需要的部分。
以下是一个简单的例子,假设我们有一个SQL查询,比如:
```java
String sql = "SELECT * FROM table WHERE column1 = 'value1' AND (column2 > 10 OR column3 = 'anotherValue')";
```
你可以使用JSqlParser的`SimpleSelect`类来解析这个查询,然后遍历`WhereElement`结构,剔除特定的条件。这里是一个大概的步骤:
```java
import net.sf.jsqlparser.JSQLParserException;
import net.sf.jsqlparser.parser.CCJSqlParserUtil;
import net.sf.jsqlparser.statement.select.Select;
import net.sf.jsqlparser.util.Column;
import net.sf.jsqlparser.util.SimpleSelect;
public void removeCondition(String conditionToRemove) {
SimpleSelect simpleSelect = null;
try {
simpleSelect = (SimpleSelect) CCJSqlParserUtil.parse(sql);
// 遍历where子句的每个元素
for (net.sf.jsqlparser.statement.select.WhereElement whereElement : simpleSelect.getWhere()) {
if (!(whereElement instanceof net.sf.jsqlparser.clause.where.AndExpression || whereElement instanceof net.sf.jsqlparser.clause.where.OrExpression)) {
continue; // 如果不是and/or表达式,直接跳过
}
AndExpression orExpression = null;
if (whereElement instanceof AndExpression) {
orExpression = (AndExpression) whereElement;
} else {
orExpression = (AndExpression) ((OrExpression) whereElement).getLeftItem();
}
// 检查条件是否是我们要移除的
boolean shouldRemove = false;
for (net.sf.jsqlparser.statement.select.Condition condition : orExpression.getItems()) {
if (condition instanceof net.sf.jsqlparser.clause.equality.Comparison) {
Comparison comparison = (Comparison) condition;
if (comparison.getRight() instanceof Column && comparison.getRight().getName().equals(conditionToRemove)) {
shouldRemove = true;
break;
}
}
}
if (!shouldRemove) {
// 如果不应该移除,保留该表达式
simpleSelect.setWhere(orExpression);
} else {
// 移除条件
andExpression.getItems().remove(whereElement);
}
}
String newSql = simpleSelect.toSqlString().getSql(); // 将处理后的SQL重新组合
System.out.println(newSql); // 输出新的查询结果,去除了指定的条件
} catch (JSQLParserException e) {
e.printStackTrace();
}
}
```
在这个例子中,我们假设你想从查询中移除`column1 = 'value1'`的条件。将`conditionToRemove`变量设置为你想移除的条件名即可。
阅读全文
相关推荐
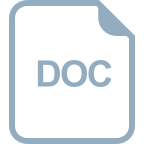
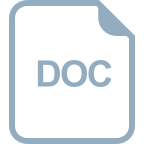
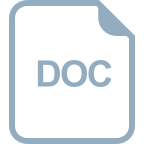


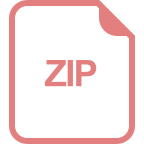

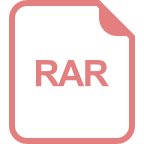
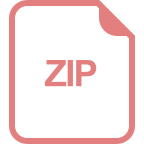
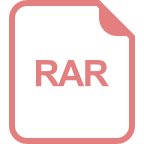
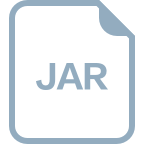
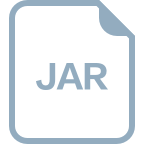