es8新特性 并举例说明
时间: 2024-02-11 18:27:04 浏览: 26
ES8(ECMAScript 2017)引入了一些新的语言特性,下面是其中一些主要的特性,并举例说明:
1. 字符串填充方法:ES8引入了字符串的`padStart`和`padEnd`方法,用于在字符串的开头或结尾插入指定的字符,以达到指定的长度。
```javascript
const str = 'Hello';
console.log(str.padStart(10, 'x')); // Output: "xxxxHello"
console.log(str.padEnd(10, 'x')); // Output: "Helloxxxxx"
```
2. 异步函数:ES8引入了`async/await`关键字,用于简化使用Promise处理异步操作的代码。`async`关键字用于定义一个异步函数,而`await`关键字用于暂停异步函数的执行,等待一个Promise解析为结果。
```javascript
function delay(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function greet() {
await delay(2000); // 等待2秒
console.log('Hello, world!');
}
greet(); // 输出: "Hello, world!"(在等待2秒后)
```
3. 共享内存和原子操作:ES8引入了`SharedArrayBuffer`和`Atomics`对象,用于支持共享内存并进行原子操作。这允许多个线程在共享内存上进行并发操作。
这里没有具体的示例代码,因为共享内存和原子操作是高级主题,需要在特定环境中使用。
4. 正则表达式命名捕获组:ES8允许给正则表达式的捕获组命名,以便更方便地引用和提取匹配的结果。
```javascript
const regex = /(?<year>\d{4})-(?<month>\d{2})-(?<day>\d{2})/;
const match = regex.exec('2022-01-01');
console.log(match.groups.year); // Output: "2022"
console.log(match.groups.month); // Output: "01"
console.log(match.groups.day); // Output: "01"
```
5. Object.getOwnPropertyDescriptors:这个静态方法返回一个对象的所有自身属性的描述符。这样可以更方便地获取对象属性的详细信息。
```javascript
const obj = {
name: 'Alice',
age: 25
};
const descriptors = Object.getOwnPropertyDescriptors(obj);
console.log(descriptors.name); // Output: { value: 'Alice', writable: true, enumerable: true, configurable: true }
console.log(descriptors.age); // Output: { value: 25, writable: true, enumerable: true, configurable: true }
```
这些是ES8引入的一些新特性,它们为开发者提供了更方便、更强大的编程工具,并进一步改善了JavaScript语言的功能和性能。
相关推荐
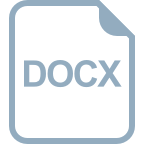














