给定一个字符串str按字符串中字符出现频率从高到低输出到列表,如果次数相同则按字母顺序排列
时间: 2024-10-09 08:09:40 浏览: 48
给定一个字符串str,按照字符串中字符出现频率从高到低输出到列表,如果次数相同则按字母顺序排列,可以按照以下步骤进行操作:
1. 首先,我们可以使用collections模块中的Counter函数来统计字符串中每个字符出现的次数,并将其存储在一个字典中。
2. 接下来,我们可以使用sorted函数对字典中的键值对进行排序,排序的依据是先按照值从大到小排序,如果值相同则按照键从小到大排序。
3. 最后,我们可以将排序后的键值对转换为一个列表,并只保留键值对中的键,即字符串中的字符。
代码实现如下:
```
import collections
def frequency_sort(s):
# 统计每个字符出现的次数
count = collections.Counter(s)
# 按照出现次数从大到小,键从小到大排序
sorted_count = sorted(count.items(), key=lambda x: (-x[1], x[0]))
# 只保留键,即字符串中的字符
result = [char * freq for char, freq in sorted_count]
# 将列表中的字符串连接起来
return ''.join(result)
```
相关问题
C语言中给定一个字符串 str,要求你输出该字符串的所有不同的排列。并按字典升序排列
在C语言中,生成一个字符串的所有不同排列通常需要借助回溯算法或者递归的方法。以下是一个简单的思路和示例代码,用于生成字符串的全排列并按照字典顺序排序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 排列函数
void permute(char *str, int l, int r) {
if (l == r)
printf("%s\n", str);
else {
for(int i = l; i <= r; i++) {
// 交换字符
char temp = str[l];
str[l] = str[i];
str[i] = temp;
// 递归处理剩余部分
permute(str, l + 1, r);
// 回溯:恢复原始字符位置
temp = str[l];
str[l] = str[i];
str[i] = temp;
}
}
}
int main() {
char str[100];
printf("Enter a string: ");
fgets(str, sizeof(str), stdin); // 注意这里要移除fgets读取的换行符
str[strlen(str)-1] = '\0'; // 结束字符串
int n = strlen(str);
sort(str, str+n); // 先对字符串进行排序
permute(str, 0, n-1);
return 0;
}
```
在这个程序中,`permute`函数采用了深度优先搜索的思想,通过不断交换字符位置生成新的排列,并在完成后恢复原始状态。先对字符串进行排序,是为了确保结果按照字典序排列。
给定一个字符串中有相同的单词,请输出该单词在整个字符串中出现的次数
要计算给定字符串中相同单词的出现次数,你可以使用Python中的内置数据结构和函数。这里我们可以先将字符串分割成单词列表,然后使用字典(dictionary)来存储每个单词及其对应的计数。
以下是一个简单的示例代码:
```python
def count_word_occurrences(input_string):
# 将字符串转换为小写并删除标点符号,以便不区分大小写和考虑标点不影响单词计数
input_string = input_string.lower().replace(",", "").replace(".", "").replace("!", "").replace("?", "")
# 分割字符串为单词列表
words_list = input_string.split()
# 创建一个空字典用于存储单词计数
word_count = {}
# 遍历单词列表,更新字典中的计数
for word in words_list:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
# 测试代码
input_str = "Hello world! Hello, this is a test. Hello again."
word_counts = count_word_occurrences(input_str)
for word, count in word_counts.items():
print(f"'{word}': {count}")
```
运行这段代码后,你会得到每个单词及其在原始字符串中出现的次数。
阅读全文
相关推荐
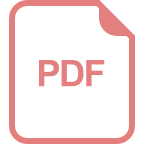
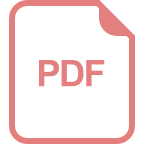
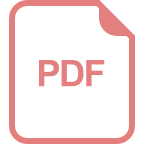













