C语言初始化单链表,并进行排序
时间: 2024-09-26 11:00:29 浏览: 5
在C语言中,初始化单链表并对其进行排序通常需要以下几个步骤:
1. **创建节点结构**:
首先,我们需要定义一个链表节点结构,包含一个数据域和指向下一个节点的指针。例如:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
2. **初始化链表**:
使用`malloc`动态分配内存创建节点,并将它们链接在一起。例如,可以编写一个函数来插入元素到链表的末尾:
```c
void insertNode(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
} else {
Node* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
```
要初始化空链表,可以设置`head`为`NULL`。
3. **排序链表**:
对于升序排列,最简单的是选择适当的排序算法,如冒泡排序、插入排序或归并排序,然后遍历整个链表应用排序算法。这里以插入排序为例:
```c
void insertionSortList(Node** head) {
Node* current, *temp;
int value;
for (current = *head; current != NULL && current->next != NULL; current = current->next) {
value = current->data;
temp = current->next;
while (temp != NULL && temp->data < value) {
current->data = temp->data;
temp = temp->next;
}
current->data = value;
}
}
```
4. **完整示例**:
这是一个完整的例子,包括创建链表和排序:
```c
// 初始化链表
Node* head = NULL;
insertNode(&head, 5);
insertNode(&head, 3);
insertNode(&head, 7);
insertNode(&head, 1);
// 排序链表
insertionSortList(&head);
相关推荐
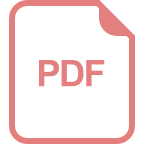
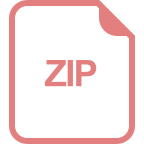
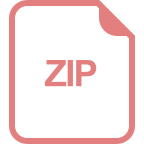














