da18b20温度传感器stm32rct6
时间: 2023-11-26 09:47:03 浏览: 28
DS18B20是一种数字温度传感器,可以通过单总线接口与微控制器通信。下面是DS18B20温度传感器在STM32单片机上的驱动软件例程源码:
```c
#include "main.h"
#include "ds18b20.h"
/* DS18B20读取温度函数 */
float DS18B20_ReadTemp(void)
{
uint8_t i, present = 0;
uint8_t data[12];
float temperature = 0;
/* 发送读取温度命令 */
DS18B20_Reset();
DS18B20_WriteByte(0xCC);
DS18B20_WriteByte(0x44);
/* 等待转换完成 */
while (!present)
{
present = DS18B20_Reset();
DS18B20_WriteByte(0xCC);
DS18B20_WriteByte(0xBE);
for (i = 0; i < 9; i++)
{
data[i] = DS18B20_ReadByte();
}
present = 1;
for (i = 0; i < 8; i++)
{
if (data[i] != 0xFF)
{
present = 0;
}
}
}
/* 计算温度值 */
temperature = (float)((data[1] << 8) | data[0]) / 16.0;
return temperature;
}
/* DS18B20复位函数 */
uint8_t DS18B20_Reset(void)
{
uint8_t presence = 0;
/* 发送复位脉冲 */
DS18B20_DQ_OUT();
DS18B20_DQ_LOW();
HAL_Delay(480);
DS18B20_DQ_IN();
HAL_Delay(80);
/* 检测DS18B20响应信号 */
if (!DS18B20_DQ_READ())
{
presence = 1;
}
HAL_Delay(400);
return presence;
}
/* DS18B20写字节函数 */
void DS18B20_WriteByte(uint8_t data)
{
uint8_t i;
DS18B20_DQ_OUT();
for (i = 0; i < 8; i++)
{
if ((data >> i) & 0x01)
{
DS18B20_DQ_LOW();
HAL_Delay(2);
DS18B20_DQ_HIGH();
HAL_Delay(60);
}
else
{
DS18B20_DQ_LOW();
HAL_Delay(60);
DS18B20_DQ_HIGH();
HAL_Delay(2);
}
}
}
/* DS18B20读字节函数 */
uint8_t DS18B20_ReadByte(void)
{
uint8_t i, data = 0;
DS18B20_DQ_OUT();
for (i = 0; i < 8; i++)
{
DS18B20_DQ_LOW();
HAL_Delay(2);
DS18B20_DQ_IN();
HAL_Delay(8);
if (DS18B20_DQ_READ())
{
data |= 0x01 << i;
}
HAL_Delay(50);
}
return data;
}
```
此外,还需要在ds18b20.h文件中定义相关的宏和函数声明:
```c
#ifndef __DS18B20_H
#define __DS18B20_H
#include "main.h"
/* DS18B20引脚定义 */
#define DS18B20_DQ_GPIO_Port GPIOA
#define DS18B20_DQ_Pin GPIO_PIN_0
/* DS18B20引脚操作宏定义 */
#define DS18B20_DQ_OUT() HAL_GPIO_WritePin(DS18B20_DQ_GPIO_Port, DS18B20_DQ_Pin, GPIO_PIN_RESET)
#define DS18B20_DQ_IN() HAL_GPIO_WritePin(DS18B20_DQ_GPIO_Port, DS18B20_DQ_Pin, GPIO_PIN_SET)
#define DS18B20_DQ_LOW() HAL_GPIO_WritePin(DS18B20_DQ_GPIO_Port, DS18B20_DQ_Pin, GPIO_PIN_RESET)
#define DS18B20_DQ_HIGH() HAL_GPIO_WritePin(DS18B20_DQ_GPIO_Port, DS18B20_DQ_Pin, GPIO_PIN_SET)
#define DS18B20_DQ_READ() HAL_GPIO_ReadPin(DS18B20_DQ_GPIO_Port, DS18B20_DQ_Pin)
/* DS18B20函数声明 */
float DS18B20_ReadTemp(void);
uint8_t DS18B20_Reset(void);
void DS18B20_WriteByte(uint8_t data);
uint8_t DS18B20_ReadByte(void);
#endif /* __DS18B20_H */
```
相关推荐
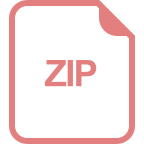
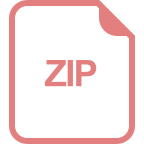
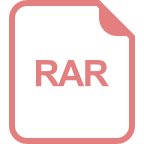
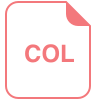
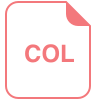
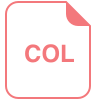
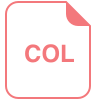
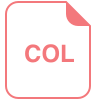









