如何用C语言编写程序来统计一个 字符串中各类型字符的数量?
时间: 2024-12-11 12:31:05 浏览: 13
在C语言中,可以使用数组来统计字符串中各类型字符(ASCII码值)的数量。这里是一个简单的示例,我们将遍历字符串并使用计数数组存储每个字符类型的频率:
```c
#include <stdio.h>
#include <string.h>
#define MAX_CHAR 128 // ASCII 码范围
// 初始化字符计数数组
int char_count[MAX_CHAR] = {0};
// 统计函数
void count_chars(char *str) {
while (*str) {
if (*str >= 'a' && *str <= 'z') { // 小写字母
char_count[*str - 'a']++;
} else if (*str >= 'A' && *str <= 'Z') { // 大写字母
char_count[*str - 'A']++;
} else if (*str >= '0' && *str <= '9') { // 数字
char_count[*str - '0']++;
} else if (*str == ' ' || *str == '\t' || *str == '\n') { // 空格、制表符、换行符
char_count[32]++;
} else { // 其他特殊字符
char_count[*str]++;
}
str++;
}
}
int main() {
char input_str[100];
printf("Enter a string: ");
fgets(input_str, sizeof(input_str), stdin); // 防止读入空字符
input_str[strlen(input_str) - 1] = '\0'; // 去掉换行符
count_chars(input_str);
for (int i = 0; i < MAX_CHAR; i++) {
if (char_count[i]) {
printf("Character '%c': %d times\n", i, char_count[i]);
}
}
return 0;
}
```
在这个程序中,我们首先初始化一个大小为128的`char_count`数组,因为ASCII码共包含128个字符。然后定义了一个`count_chars`函数,通过遍历输入的字符串,对每个字符进行分类统计。最后,在`main`函数里打印出每个字符及其出现次数。
阅读全文
相关推荐
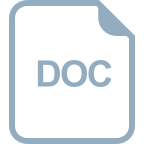
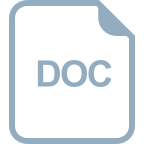
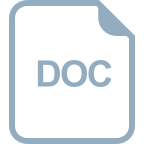
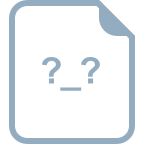
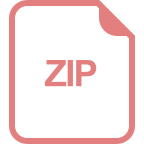

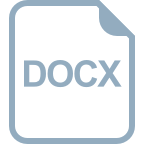
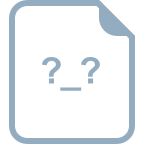
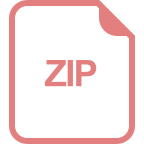
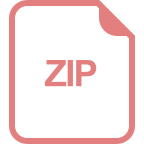
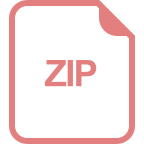
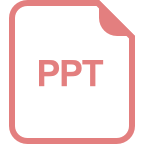
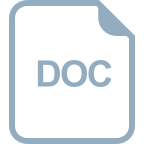
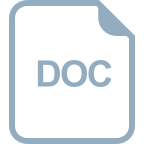
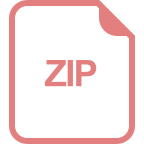