C++字节流与结构体
时间: 2024-12-12 07:14:14 浏览: 11
在C++编程中,字节流和结构体是两个常见且重要的概念。它们在数据处理和网络通信中经常被使用。
### 字节流
字节流(Byte Stream)是指一系列连续的字节数据。在C++中,字节流通常通过`std::istream`和`std::ostream`类来操作。字节流可以用于文件读写、网络通信等场景。
#### 示例代码
```cpp
#include <iostream>
#include <fstream>
#include <vector>
int main() {
// 写入字节流到文件
std::ofstream outFile("example.bin", std::ios::binary);
std::vector<char> data = {'H', 'e', 'l', 'l', 'o'};
outFile.write(data.data(), data.size());
outFile.close();
// 从文件读取字节流
std::ifstream inFile("example.bin", std::ios::binary);
std::vector<char> readData(data.size());
inFile.read(readData.data(), readData.size());
inFile.close();
// 输出读取的数据
for (char c : readData) {
std::cout << c;
}
std::cout << std::endl;
return 0;
}
```
### 结构体
结构体(Struct)是C++中的一种用户自定义数据类型,用于将不同类型的数据组合在一起。结构体在处理复杂数据时非常有用。
#### 示例代码
```cpp
#include <iostream>
#include <fstream>
struct Person {
char name[50];
int age;
float height;
};
int main() {
// 创建一个结构体实例
Person person = {"Alice", 30, 1.65f};
// 将结构体写入文件
std::ofstream outFile("person.bin", std::ios::binary);
outFile.write(reinterpret_cast<char*>(&person), sizeof(Person));
outFile.close();
// 从文件读取结构体
Person readPerson;
std::ifstream inFile("person.bin", std::ios::binary);
inFile.read(reinterpret_cast<char*>(&readPerson), sizeof(Person));
inFile.close();
// 输出读取的结构体数据
std::cout << "Name: " << readPerson.name << std::endl;
std::cout << "Age: " << readPerson.age << std::endl;
std::cout << "Height: " << readPerson.height << std::endl;
return 0;
}
```
### 字节流与结构体的结合
在实际应用中,字节流和结构体经常结合使用。例如,可以通过字节流将结构体数据写入文件或通过网络传输。
#### 示例代码
```cpp
#include <iostream>
#include <fstream>
struct Person {
char name[50];
int age;
float height;
};
int main() {
// 创建结构体实例并写入字节流
Person person = {"Bob", 25, 1.75f};
std::ofstream outFile("person.bin", std::ios::binary);
outFile.write(reinterpret_cast<char*>(&person), sizeof(Person));
outFile.close();
// 从字节流中读取结构体数据
Person readPerson;
std::ifstream inFile("person.bin", std::ios::binary);
inFile.read(reinterpret_cast<char*>(&readPerson), sizeof(Person));
inFile.close();
// 输出读取的结构体数据
std::cout << "Name: " << readPerson.name << std::endl;
std::cout << "Age: " << readPerson.age << std::endl;
std::cout << "Height: " << readPerson.height << std::endl;
return 0;
}
```
通过以上示例代码,可以看出字节流和结构体在C++中的应用非常广泛。字节流可以用于数据的序列化和反序列化,而结构体则用于组织复杂的数据。
阅读全文
相关推荐
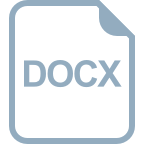
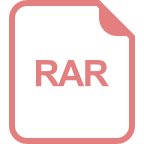
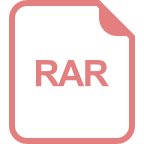

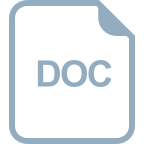



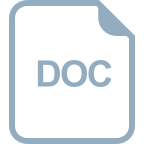
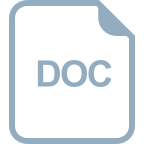
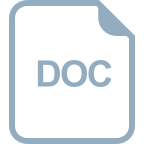
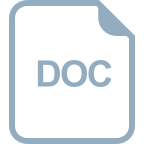
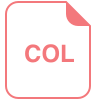





