本题要求编写程序,读入5个字符串,按由小到大的顺序输出。 输入为由空格分隔的5个非空字符串,每个字符串不包括空格、制表符、换行符等空白字符,长度小于80。
时间: 2024-12-28 18:30:31 浏览: 18
要实现这个功能,你可以使用C语言中的`scanf`函数来读取输入的字符串,然后创建一个结构体来存储这些字符串并附带一个比较函数用于排序。这里有一个简单的示例程序:
```c
#include <stdio.h>
#include <string.h>
// 定义一个结构体来存储字符串及其索引
typedef struct {
char str[81];
int index;
} StringWithIndex;
// 比较两个字符串的大小
int compare_strings(const void *a, const void *b) {
const StringWithIndex *sa = (const StringWithIndex *)a;
const StringWithIndex *sb = (const StringWithIndex *)b;
return strcmp(sa->str, sb->str); // 使用strcmp进行字典序比较
}
int main() {
// 声明数组用于存储字符串及其索引
StringWithIndex strings[5];
// 读取并保存5个字符串
for (int i = 0; i < 5; i++) {
fgets(strings[i].str, sizeof(strings[i].str), stdin);
strings[i].index = i; // 添加索引以便在排序后知道原来的相对位置
strings[i].str[strcspn(strings[i].str, " \t\n")] = '\0'; // 移除末尾的空白字符
}
// 对字符串数组进行排序
qsort(strings, 5, sizeof(StringWithIndex), compare_strings);
// 输出排序后的字符串
for (int i = 0; i < 5; i++) {
printf("%s (index %d)\n", strings[i].str, strings[i].index);
}
return 0;
}
```
在这个程序中,我们首先定义了一个结构体`StringWithIndex`,包含字符串和对应的索引。接着,我们提供了一个自定义的比较函数`compare_strings`,它根据`strcmp`函数对字符串进行比较。
在`main`函数中,我们读取5个字符串,存储在`strings`数组中,并用`fgets`确保移除了末尾的空白字符。然后,我们调用`qsort`函数对数组进行排序,最后遍历并输出已排序的字符串。
运行此程序时,按照题目要求,从标准输入(通常是键盘)逐个输入5个字符串,它们就会被自动按字典序从小到大排列并输出。
阅读全文
相关推荐
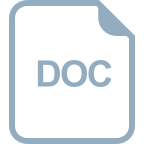
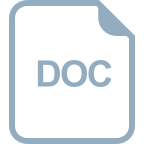
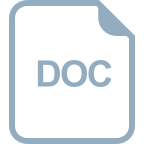















