ArrayList数组赋值
时间: 2023-10-24 07:09:12 浏览: 162
要为ArrayList数组赋值,可以使用add()方法将元素添加到数组中。例如,下面的代码展示了如何给一个ArrayList数组赋值:
```java
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
ArrayList<String> arrayList = new ArrayList<>();
// 使用add()方法给数组赋值
arrayList.add("元素1");
arrayList.add("元素2");
arrayList.add("元素3");
System.out.println(arrayList);
}
}
```
上述代码创建了一个ArrayList对象并命名为arrayList。然后,使用add()方法将三个字符串元素添加到数组中。最后,使用System.out.println()语句打印出ArrayList数组的内容。
运行上述代码,将输出:
```
[元素1, 元素2, 元素3]
```
这表示成功给ArrayList数组赋值。
相关问题
将List数组赋值给list数组
在Java中,如果你有一个List类型的数组(例如 List<Integer>[] 或者 List<String>[]),并且你想将其赋值给另一个List数组,通常需要逐个元素地复制。因为原始的List数组通常不是直接可以转换到List数组的。这里是一个简单的示例:
```java
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
// 假设我们有原始的List数组
List<Integer> list1 = Arrays.asList(1, 2, 3);
List<Integer> list2 = Arrays.asList(4, 5, 6);
List<Integer>[] originalLists = new List[2];
originalLists[0] = list1;
originalLists[1] = list2;
// 创建一个新的List数组来接收数据
List<Integer>[] newList = new List[2];
// 逐个复制数据到新的List数组
for (int i = 0; i < originalLists.length; i++) {
newList[i] = new ArrayList<>(originalLists[i]); // 使用ArrayList拷贝
}
// 输出新数组的内容以验证
for (List<Integer> sublist : newList) {
System.out.println(sublist);
}
}
}
```
在上述代码中,我们先创建了一个包含两个List的原始列表数组,然后创建了一个新的空列表数组。接着遍历原数组,将每个元素的引用复制到新数组中。这样就完成了从List数组到List数组的赋值。
grpc protoc3数组赋值repeated NetDevice NetMsg = 1; request赋值
在gRPC协议中,`protoc3` 是 Protocol Buffers 的一种生成工具,用于将.proto(Protocol Buffer 定义文件)转换为不同的语言支持的源代码。当你看到 `repeated NetDevice NetMsg = 1;` 这样的定义,它表示 `NetMsg` 是一个包含多个 `NetDevice` 对象的数组。`NetDevice` 可能是一个自定义的数据结构,包含了你需要在网络请求中发送的信息。
如果你想要在 gRPC 请求(request)中设置这个字段,通常你会这样做:
1. 首先,在客户端代码里,创建一个 `NetDevice` 的列表或数组:
```python
// 如果 NetDevice 是一个 Python 类
net_devices = [NetDevice(device_id=1, device_name="DeviceA"), NetDevice(device_id=2, device_name="DeviceB")]
// 或者如果是 Java,可以这样初始化:
List<NetDevice> netDevices = new ArrayList<>();
netDevices.add(new NetDevice(1, "DeviceA"));
netDevices.add(new NetDevice(2, "DeviceB"));
```
2. 然后,将这个列表赋值给 `NetMsg` 字段:
```python
net_msg_request = NetMsgRequest()
net_msg_request.net_msgs.extend(net_devices) # Python
net_msg_request.set_net_msgs(net_devices); // Java (假设有set_net_msgs方法)
```
这里的 `.extend()` 或 `set_net_msgs` 方法会将整个列表复制到protobuf消息的相应字段中。
阅读全文
相关推荐
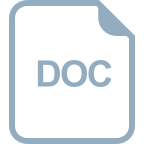
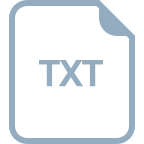
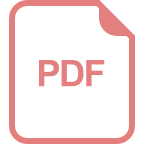
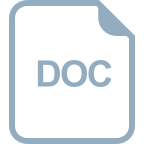
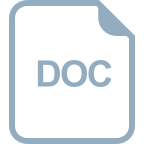

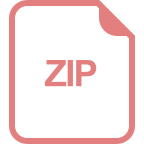
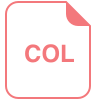








