nodejs+讯飞实现在线语音合成
时间: 2023-11-18 21:04:28 浏览: 125
要在 Node.js 中实现讯飞的在线语音合成功能,你可以使用科大讯飞开放平台提供的语音合成接口。以下是一个示例代码,演示如何使用 Node.js 发送 HTTP 请求调用讯飞语音合成接口:
```javascript
const fs = require('fs');
const crypto = require('crypto');
const request = require('request');
const appid = 'your_appid'; // 替换为你的 AppID
const apiSecret = 'your_api_secret'; // 替换为你的 API Secret
const apiKey = 'your_api_key'; // 替换为你的 API Key
const text = '要合成的文本内容'; // 替换为你要合成的文本内容
// 构造请求头
const curTime = Math.floor(Date.now() / 1000).toString();
const param = {
auf: 'audio/L16;rate=16000',
aue: 'lame',
voice_name: 'xiaoyan',
speed: '50',
volume: '50',
pitch: '50',
engine_type: 'intp65',
text_type: 'text',
};
const paramStr = JSON.stringify(param);
const checkSum = crypto
.createHash('md5')
.update(apiKey + curTime + paramStr)
.digest('hex');
const headers = {
'Content-Type': 'application/x-www-form-urlencoded; charset=utf-8',
'X-Appid': appid,
'X-CurTime': curTime,
'X-Param': paramStr,
'X-CheckSum': checkSum,
};
// 发起请求
const options = {
url: 'http://api.xfyun.cn/v1/service/v1/tts',
method: 'POST',
headers: headers,
body: 'text=' + encodeURIComponent(text),
};
request(options, (error, response, body) => {
if (!error && response.statusCode == 200) {
fs.writeFileSync('output.mp3', body, 'binary'); // 将合成的音频保存为文件
console.log('语音合成成功');
} else {
console.error(error);
}
});
```
在上面的示例代码中,你需要将 `your_appid`、`your_api_secret`、`your_api_key` 和 `要合成的文本内容` 替换为你自己的 AppID、API Secret、API Key 和要合成的文本。代码会将文本发送给讯飞语音合成接口,返回合成后的音频数据,并将其保存为名为 `output.mp3` 的文件。
请确保已安装 `request` 模块,你可以使用以下命令进行安装:
```
npm install request
```
需要注意的是,科大讯飞开放平台还有其他接口和功能可供使用,你可以根据自己的需求调用相应的接口。详细的接口文档和使用说明可以在科大讯飞开放平台官网上找到。
阅读全文
相关推荐
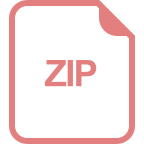
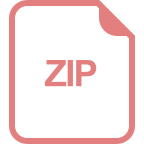
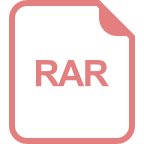
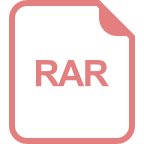
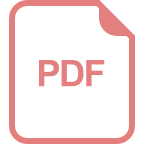
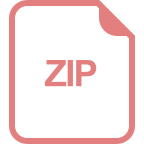
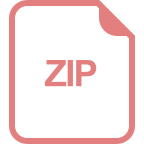
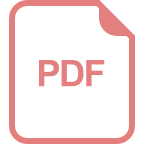
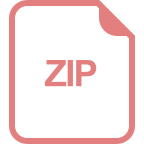
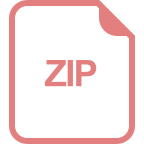
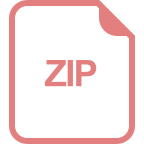
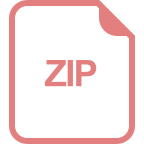
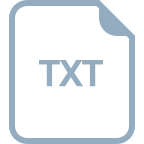
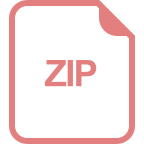
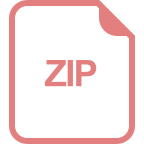
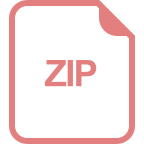