写一个多线程c++安全的单例,使用锁实现,双重检查
时间: 2024-09-15 10:15:24 浏览: 39
在C++中,为了保证线程安全地实现单例模式,我们可以利用双重检查锁定(DCL)的技术,并配合互斥锁(mutex)来防止竞态条件。下面是一个简单的示例:
```cpp
#include <mutex>
class Singleton {
private:
// 初始化静态变量和mutex
static std::once_flag flag;
static std::mutex mtx;
static std::shared_ptr<Singleton> instance;
protected:
// 防止外部直接访问构造函数
Singleton() {}
public:
// 单例获取方法
static std::shared_ptr<Singleton>& getInstance() {
std::call_once(flag, []() {
std::lock_guard<std::mutex> lock(mtx);
if (!instance)
instance = std::make_shared<Singleton>();
});
return *instance;
}
};
// 初始化标志和互斥锁
std::once_flag Singleton::flag;
std::mutex Singleton::mtx;
std::shared_ptr<Singleton> Singleton::instance = nullptr;
```
在这个例子中,我们首先通过`std::once_flag`保证代码块只会被执行一次,然后使用`std::lock_guard`来自动管理对`std::mutex`的锁定,确保在任何时候只有一个线程能进入`getInstance`方法。只有在第一次调用时才会创建单例实例,之后的调用将直接返回已经存在的实例。
相关推荐
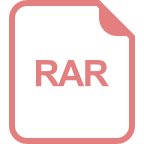
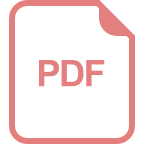
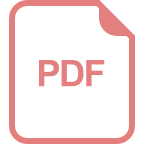













