C#语言写一个软件安装包程序,该安装包部署在服务器上特定的文件夹上,软件版本更新,软件弹出提醒对话框,点击对话框完成升级,请给出以上的叙述的详细源码
时间: 2024-12-12 09:22:01 浏览: 8
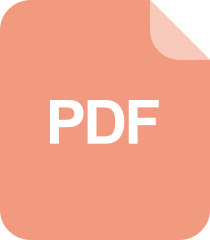
C#实现在前端网页弹出警告对话框(alert)的方法
在C#中,创建一个简单的软件安装包并处理版本更新及升级提示通常涉及到Windows Installer(MSI)技术。以下是一个简化的示例,展示了如何使用WiX (Windows Installer XML) 来创建基本的安装包,并使用WPF来显示升级提醒。请注意,这只是一个基础框架,实际项目可能会更复杂,包括错误处理、进度指示等。
首先,安装项目的WiX脚本(`Install.wxs`):
```xml
<?xml version="1.0" encoding="utf-8"?>
<Wix xmlns="http://schemas.microsoft.com/wix/2006/wi">
<Product Id="*" Name="YourSoftware" Version="1.0.0" Manufacturer="YourName" UpgradeCode="{GUID}">
<Package InstallerVersion="200" Compressed="yes" />
<Directory Id="TARGETDIR" Name="SourceDir">
<Directory Id="INSTALL_DIR" Name="YourAppFolder">
<!-- 将你的软件复制到此处 -->
<Component Id="SoftwareComponent" Guid="...">
<File Id="SoftwareFile" Source="YourSoftwareSetup.exe" KeyPath="yes" />
</Component>
</Directory>
<!-- 添加升级逻辑 -->
<Property Id="CURRENT_VERSION" Value="[产品版本]" />
<Upgrade Id="{GUID}">
<UpgradeStep Id="CheckCurrentVersion" After="CostFinalize">
<Property Action="check">NEWERVERSIONDETECTED</Property>
</UpgradeStep>
<UpgradeStep Id="PromptForUpdate" Message="A new version of the software is available. Click to upgrade." RequiresUserAction="yes" />
<UpgradeStep Id="DoUpdate" After="PromptForUpdate">
<Property Action="launch">SOFTWAREFILE</Property>
</UpgradeStep>
</Upgrade>
<!-- 如果检测到新版本 -->
<Property Id="NEWERVERSIONDETECTED" Value="YOUR_CURRENT_VERSION!=CURRENT_VERSION" />
</Directory>
</Product>
</Wix>
```
然后,创建一个简单的WPF应用程序(例如 `UpgradePrompt.xaml.cs`)用于显示升级提示:
```csharp
using System.Windows;
using System.Windows.Controls;
public partial class UpgradePrompt : Window
{
public UpgradePrompt()
{
InitializeComponent();
// 这里可以添加UI元素,如MessageBox.Show() 或者 MessageBox.ShowAsync() 提示升级
if (Environment.GetFolderPath(Environment.SpecialFolder.ApplicationData).Contains("UpgradeDetected"))
{
var message = "A newer version is available. Click to upgrade.";
MessageBox.Show(message);
}
}
private void Button_Click(object sender, RoutedEventArgs e)
{
// 调用实际的升级过程
Process.Start("your-software-updater.exe");
Close();
}
}
```
最后,创建一个启动器应用程序(如 `InstallerLauncher.exe`),它会检查是否有新版本并启动升级过程:
```csharp
using System.Diagnostics;
namespace InstallerLauncher
{
class Program
{
static void Main(string[] args)
{
// 检查是否需要升级
string currentVersion = "1.0.0"; // 你的当前软件版本
if (currentVersion != GetCurrentVersion()) // 替换为获取实际版本的方式
{
// 显示升级提示窗口
var upgradePrompt = new UpgradePrompt();
upgradePrompt.ShowDialog();
// 点击升级后运行升级程序
Process.Start("your-software-updater.exe");
}
else
{
// 直接运行软件
Process.Start("YOUR_APP_EXE_PATH");
}
}
private static string GetCurrentVersion() { /* 获取实际版本代码 */ }
}
}
```
阅读全文
相关推荐
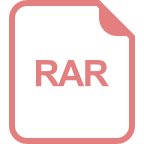
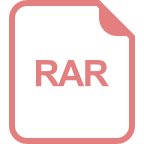
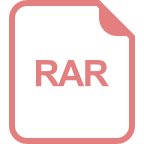
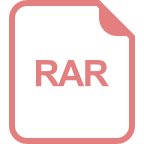
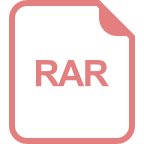
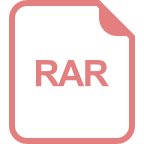
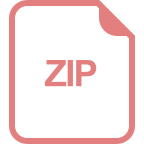
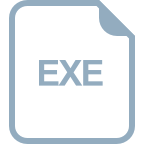
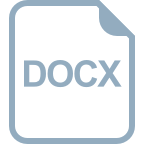
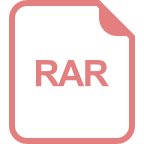
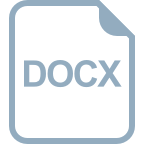
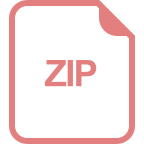
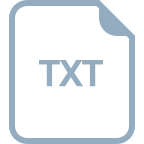
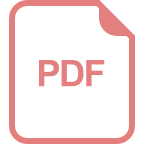
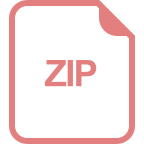
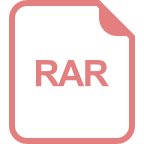
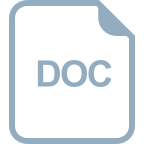