在visual studio创建一个cuda c程序,会有一个默认的kernel.cu文件,运行该文件并观察结果
时间: 2024-10-11 10:17:24 浏览: 22
在Visual Studio中创建CUDA C程序,首先你需要安装CUDA工具包,它通常包含了一个名为CUDA C++的项目模板。以下是步骤:
1. **设置环境**:
- 打开Visual Studio,选择"创建新项目",然后在"Visual C++"类别下找到"CUDA C/C++"项。
- 选择"空白项目"或"CUDA Console Application",并输入项目名称。
2. **添加kernel.cu文件**:
- 在项目资源管理器中右键点击项目,选择"添加">>"新建项",然后在CUDA C++文件夹下,添加一个名为"kernel.cu"的新Cuda源文件。
3. **编写CUDA kernel**:
- 在`kernel.cu`中,使用CUDA编程模型编写CUDA函数,即kernel(内核),这通常是计算密集型的函数,用于执行在GPU上并行处理的任务。例如:
```c++
__global__ void myKernel(float* d_out, float* d_in, int size) {
int index = threadIdx.x + blockIdx.x * blockDim.x;
if (index < size)
d_out[index] = d_in[index] * 2.0f;
}
```
4. **链接和运行**:
- 在主机代码中,你需要将内核函数加载到设备,并指定数据传输以及执行任务的规模:
```c++
dim3 threadsPerBlock(512);
dim3 numBlocks((size + threadsPerBlock.x - 1) / threadsPerBlock.x);
cudaMalloc(&d_out, size * sizeof(float));
cudaMemcpy(d_out, in, size * sizeof(float), cudaMemcpyHostToDevice);
myKernel<<<numBlocks, threadsPerBlock>>>(d_out, d_in, size);
cudaMemcpy(out, d_out, size * sizeof(float), cudaMemcpyDeviceToHost);
```
5. **构建和调试**:
- 点击菜单栏的"Build" -> "Build Solution" 或者按F7键,编译CUDA代码。如果一切顺利,会在解决方案资源管理器的Debug目录下生成可执行文件。
- 运行这个可执行文件,查看输出结果,检查是否达到预期的效果。
6. **注意事项**:
- 使用CUDA需要对GPU内存管理和错误处理有深入理解。
- Visual Studio可能会有一些CUDA特定的配置步骤,如添加CUDA Toolkit路径等。
阅读全文
相关推荐
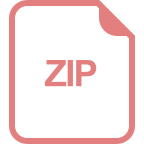
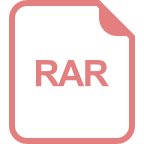
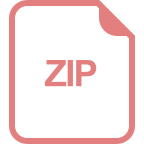
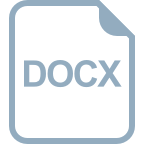
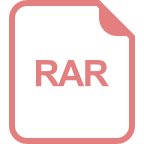
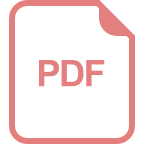
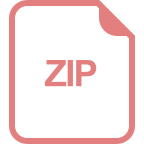
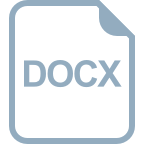
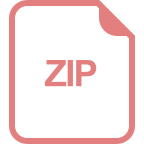
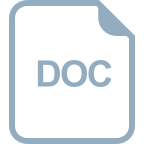
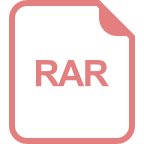
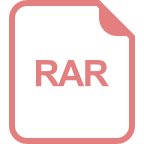
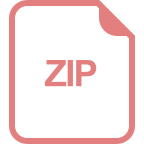
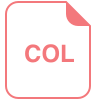
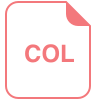

