Android studio的qq登录页面制作
时间: 2024-06-13 13:08:18 浏览: 83
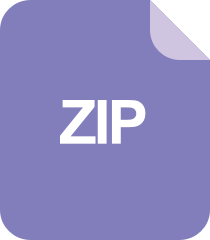
AndroidStudio实现QQ登录
以下是Android Studio实现QQ登录页面的步骤:
1.创建一个新项目并在布局文件中添加所需的UI元素,例如EditText、Button和ImageView等。
2.使用RelativeLayout或ConstraintLayout等布局管理器来设置UI元素的位置和大小。
3.在res/drawable文件夹中添加所需的图片资源,例如QQ图标和背景图片等。
4.在res/values/colors.xml文件中定义所需的颜色资源,例如按钮的背景颜色和文本颜色等。
5.在res/values/strings.xml文件中定义所需的字符串资源,例如按钮的文本和EditText的提示文本等。
6.在Java代码中实现登录逻辑,例如验证用户输入的用户名和密码是否正确等。
7.在AndroidManifest.xml文件中添加所需的权限,例如INTERNET权限和ACCESS_NETWORK_STATE权限等。
以下是一个简单的示例代码,用于实现QQ登录页面:
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/logo"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginTop="50dp"
android:src="@drawable/qq_logo" />
<EditText
android:id="@+id/username"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/logo"
android:layout_marginLeft="30dp"
android:layout_marginRight="30dp"
android:layout_marginTop="50dp"
android:hint="@string/username_hint"
android:inputType="text" />
<EditText
android:id="@+id/password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/username"
android:layout_marginLeft="30dp"
android:layout_marginRight="30dp"
android:layout_marginTop="20dp"
android:hint="@string/password_hint"
android:inputType="textPassword" />
<Button
android:id="@+id/login"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/password"
android:layout_marginLeft="30dp"
android:layout_marginRight="30dp"
android:layout_marginTop="50dp"
android:background="@color/button_background"
android:text="@string/login"
android:textColor="@color/button_text" />
</RelativeLayout>
```
```java
public class LoginActivity extends AppCompatActivity {
private EditText mUsername;
private EditText mPassword;
private Button mLogin;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
mUsername = findViewById(R.id.username);
mPassword = findViewById(R.id.password);
mLogin = findViewById(R.id.login);
mLogin.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String username = mUsername.getText().toString();
String password = mPassword.getText().toString();
// TODO: 实现登录逻辑
}
});
}
}
```
阅读全文
相关推荐
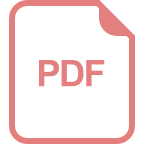
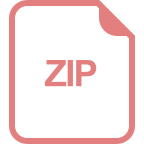

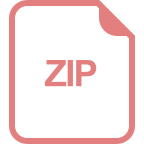



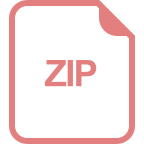
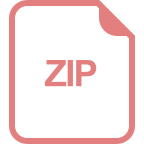
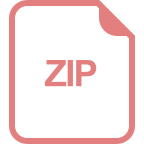
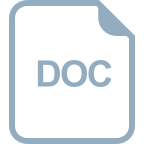
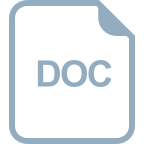

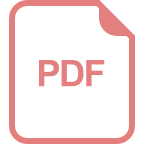
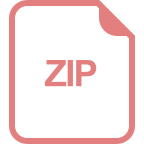
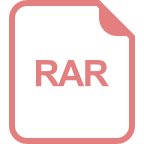
