C#中 调用Kernel32.dll读取INI文件中当前节点的所有的key和value
时间: 2024-10-23 21:21:10 浏览: 37
在C#中直接调用Windows API(如Kernel32.dll)来读取ini文件需要使用PInvoke(Platform Invoke),这涉及到底层的函数调用和内存管理。这里提供一个基本的例子,使用UnmanagedExports库简化对kernel32.dll中ReadPrivateProfileSectionEx方法的访问:
首先,你需要安装UnmanagedExports NuGet包,然后将需要暴露的函数添加到一个静态类,例如`IniReader`:
```csharp
using System;
using UnmanagedExports;
public static class IniReader
{
[DllExport("GetIniEntries", CallingConvention = CallingConvention.Cdecl)]
public static unsafe IntPtr GetIniEntries([MarshalAs(UnmanagedType.LPStr)] string filePath, [MarshalAs(UnmanagedType.LPStr)] string section, out uint size)
{
// 实际读取ini文件的代码
// 这里仅作示例,实际操作会更复杂
string[] entries = ReadIniFile(filePath, section);
if (entries != null)
{
byte* buffer = stackalloc byte[entries.Length * (sizeof(string) + sizeof(char))];
fixed (char* pChars = entries[0])
{
for (int i = 0; i < entries.Length; ++i)
{
Marshal.StringToHGlobalAnsi(pChars, ref buffer[i * (sizeof(string) + sizeof(char))]);
}
}
size = (uint)buffer.Length;
return buffer;
}
else
{
size = 0;
return IntPtr.Zero;
}
}
private static string[] ReadIniFile(string filePath, string section)
{
// 使用IniParser或其他库解析ini文件
// 示例:
var ini = IniParser.Parse(filePath);
var keys = ini.Sections[section].Keys;
return keys.Select(k => $"{k}={ini.Sections[section][k]}").ToArray();
}
}
```
注意:这只是一个简化的例子,实际操作中需要处理错误、异常,并可能需要使用DllImport关键字来声明所需函数和类型。另外,这个方法返回的是字符串数组的地址,你需要自己负责释放内存。
调用这个函数的方式:
```csharp
unsafe
{
uint size;
IntPtr ptr = IniReader.GetIniEntries(@"path\to\your.ini", "YourSection", out size);
if (ptr != IntPtr.Zero)
{
char* buffer = (char*)ptr.ToPointer();
string[] keyValuePairs = new string[size / (sizeof(string) + sizeof(char))];
for (int i = 0; i < keyValuePairs.Length; i++)
{
keyValuePairs[i] = Marshal.PtrToStringAnsi(buffer + i * (sizeof(string) + sizeof(char)));
}
// 使用keyValuePairs数组
// ...
Marshal.FreeHGlobal(ptr);
}
}
```
阅读全文
相关推荐




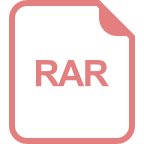

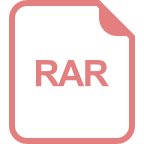
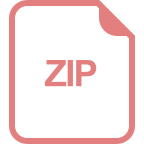




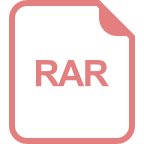


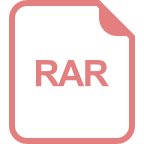
