springboot自定义注解例子
时间: 2023-11-07 08:03:50 浏览: 76
SpringBoot提供了很多方便的方式来自定义注解。一个例子是通过使用Spring AOP和Java反射来实现自定义注解校验参数。在源码中,可以在DemoController类中找到使用该注解的示例。
示例代码如下:
```java
@RestController
public class DemoController {
@GetMapping("/hello")
@CheckParams(notNull = true)
public String sayHello(@RequestParam("name") String name) {
return "Hello, " + name + "!";
}
}
```
在上述代码中,我们给`sayHello`方法添加了一个自定义注解`@CheckParams`,并将`notNull`属性设置为`true`,表示`name`参数不能为空。当请求`/hello`接口时,如果请求参数`name`为空,则会抛出异常。
相关推荐
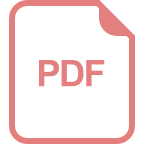
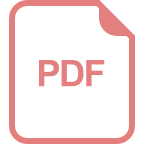
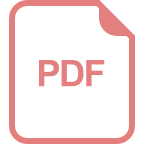
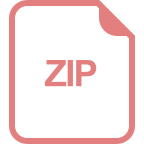
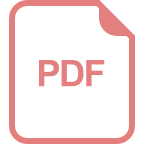
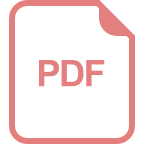
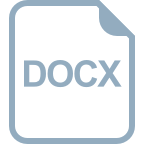
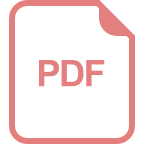
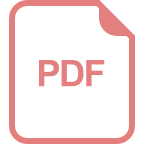
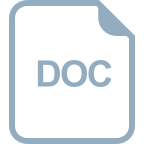
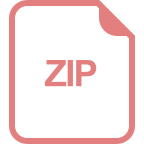
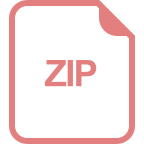
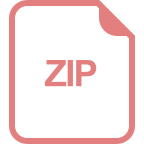
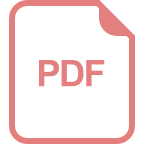
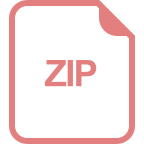