用python完成任务点二中的图像拼接功能
时间: 2024-12-17 11:22:10 浏览: 2
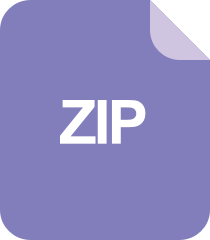
python+OpenCV实现全景图像拼接和图像黑边处理

任务点二中的图像拼接功能可以通过OpenCV实现。以下是使用Python和OpenCV进行图像拼接的详细步骤:
### 步骤概述
1. **读取图像**:加载需要拼接的两张图像。
2. **特征提取**:使用ORB(Oriented FAST and Rotated BRIEF)或SIFT(Scale-Invariant Feature Transform)等算法提取图像的关键点和描述符。
3. **特征匹配**:使用FLANN(Fast Library for Approximate Nearest Neighbors)或其他匹配器找到两幅图像之间的匹配点。
4. **单应性矩阵计算**:利用匹配点计算单应性矩阵。
5. **图像拼接**:使用单应性矩阵将两幅图像拼接在一起。
### 代码示例
```python
import cv2
import numpy as np
def stitch_images(img1_path, img2_path):
# 读取图像
img1 = cv2.imread(img1_path)
img2 = cv2.imread(img2_path)
# 初始化ORB特征检测器
orb = cv2.ORB_create()
# 检测关键点和计算描述符
keypoints1, descriptors1 = orb.detectAndCompute(img1, None)
keypoints2, descriptors2 = orb.detectAndCompute(img2, None)
# 使用FLANN进行特征匹配
index_params = dict(algorithm=6, trees=5)
search_params = dict(checks=50)
flann = cv2.FlannBasedMatcher(index_params, search_params)
matches = flann.knnMatch(descriptors1, descriptors2, k=2)
# 过滤匹配点
good_matches = []
for m, n in matches:
if m.distance < 0.7 * n.distance:
good_matches.append(m)
# 提取匹配点的坐标
src_pts = np.float32([keypoints1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([keypoints2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
# 计算单应性矩阵
H, _ = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 获取图像尺寸
h1, w1 = img1.shape[:2]
h2, w2 = img2.shape[:2]
# 计算拼接后的图像尺寸
result_shape = (w1 + w2, max(h1, h2))
# 拼接图像
result = cv2.warpPerspective(img1, H, result_shape)
result[0:h2, 0:w2] = img2
return result
# 调用函数
img1_path = 'path_to_image1.jpg'
img2_path = 'path_to_image2.jpg'
result = stitch_images(img1_path, img2_path)
# 显示结果
cv2.imshow('Stitched Image', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
### 说明
1. **读取图像**:使用`cv2.imread`读取图像文件。
2. **特征提取**:使用ORB特征检测器提取关键点和描述符。
3. **特征匹配**:使用FLANN匹配器找到两幅图像之间的匹配点,并过滤掉不好的匹配点。
4. **单应性矩阵计算**:使用`cv2.findHomography`计算单应性矩阵。
5. **图像拼接**:使用`cv2.warpPerspective`将第一张图像变形到新的视角,并将其与第二张图像拼接在一起。
通过以上步骤,你可以实现图像拼接功能。希望这段代码对你有所帮助!
阅读全文
相关推荐
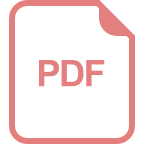
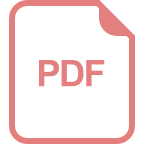
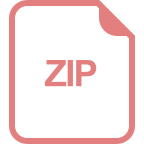
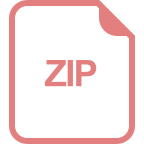
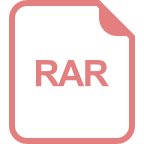
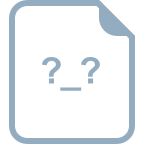
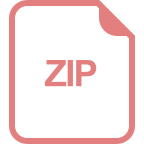
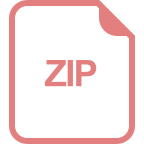
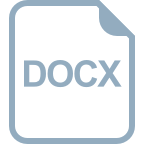
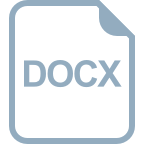
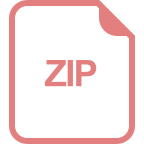
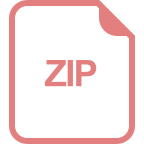
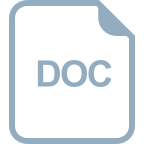
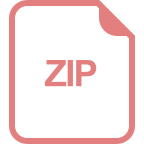
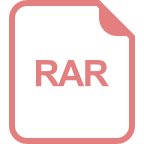