POST传入和GET传入参数的方式不同
时间: 2024-10-20 16:13:40 浏览: 16
POST和GET是HTTP请求常用的两种方法,它们在传输数据时有明显的区别:
1. **GET**: GET方法通常用于获取资源,它的参数信息会附加在URL的查询字符串部分,也就是?后面的部分。GET方法发送的数据量相对较小,并且数据会被公开显示在浏览器地址栏,不适合敏感信息的传递,因为这些信息可能会被缓存或记录。
示例:
```
http://example.com/api?param1=value1¶m2=value2
```
2. **POST**: POST方法主要用于向服务器提交数据,特别是提交大量或复杂的数据。数据不会直接暴露在URL中,而是包含在请求体(Request Body)中,这样可以保护数据的安全性。POST请求不会出现在浏览器历史记录中,也不会被缓存。
示例:
```
POST /api HTTP/1.1
Content-Type: application/x-www-form-urlencoded
Data: param1=value1¶m2=value2
```
**相关问题--:**
1. POST方法的优势是什么?
2. GET和POST如何区分参数是否安全?
3. 何时会选择使用POST而非GET?
相关问题
httpurlconnection中setRequestMethod传入post,但是还是get请求
在Java中,`HttpURLConnection` 类是用于发送HTTP请求并读取响应的一个工具。如果在使用`setRequestMethod`方法时传入了"POST",但实际上还是发出了GET请求,可能的原因有以下几点:
1. 设置请求方法的时机不正确。应该在`connect()`方法调用之前设置请求方法,如果在`connect()`之后修改,那么设置将不会生效。
2. 网络连接未正确关闭。如果之前已经进行了一个GET请求,那么可能没有正确关闭该连接,导致后续的设置被忽略。
3. 代码中存在bug,比如在设置请求方法后又不小心重置为GET。
以下是一个使用`HttpURLConnection`设置为POST请求的正确示例:
```java
URL url = new URL("http://example.com/api/resource");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setDoOutput(true);
// 写入POST请求体数据
try (OutputStream os = conn.getOutputStream()) {
byte[] input = "请求体数据".getBytes(StandardCharsets.UTF_8);
os.write(input, 0, input.length);
}
// 获取响应码,确认请求已发送
int responseCode = conn.getResponseCode();
// 处理响应...
conn.disconnect(); // 关闭连接
```
确保在`connect()`方法之前设置了请求方法,并在发送请求数据前检查是否已经开启了输出流。
帮我写一个Java的http请求工具类,包含Post和Get请求,并且支持传入header,也可以不传header等西悉尼
好的,我可以帮您解决。这里是一个示例Java的http请求工具类,它可以支持Post和Get请求,并且可以传入header或不传入header:
```
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpUtils {
public static String sendGet(String url, String headers) throws Exception {
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
// 设置请求头
if (headers != null && headers.trim().length() > 0) {
String[] headerArray = headers.split("\n");
for (String header : headerArray) {
String[] parts = header.split(":");
con.setRequestProperty(parts[0].trim(), parts[1].trim());
}
}
// 发送GET请求
con.setRequestMethod("GET");
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return response.toString();
}
// 发送Post请求
public static String sendPost(String url, String headers, String requestPayload) throws Exception {
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
// 设置请求头
if (headers != null && headers.trim().length() > 0) {
String[] headerArray = headers.split("\n");
for (String header : headerArray) {
String[] parts = header.split(":");
con.setRequestProperty(parts[0].trim(), parts[1].trim());
}
}
// 发送POST请求
con.setRequestMethod("POST");
con.setDoOutput(true);
con.getOutputStream().write(requestPayload.getBytes("UTF-8"));
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return response.toString();
}
}
```
使用示例:
```
// 发送GET请求
String url = "http://www.example.com/getData";
String headers = "Content-Type: application/json\nAuthorization: Bearer abcdefg";
String response = HttpUtils.sendGet(url, headers);
// 发送POST请求
String url = "http://www.example.com/postData";
String headers = "Content-Type: application/json\nAuthorization: Bearer abcdefg";
String requestPayload = "{\"key1\":\"value1\",\"key2\":\"value2\"}";
String response = HttpUtils.sendPost(url, headers, requestPayload);
```
请注意,此示例仅供参考。在实际编写代码过程中,还需要考虑错误处理、性能优化等方面的问题。
阅读全文
相关推荐
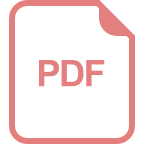
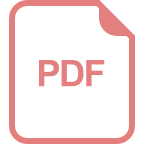
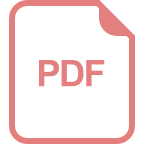
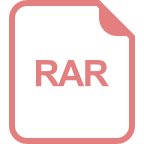
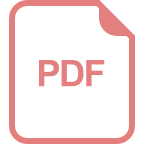
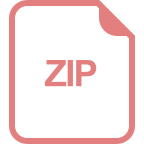
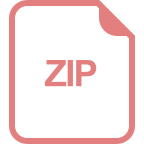
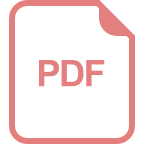
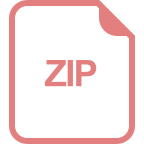
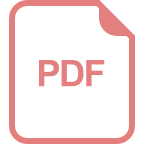
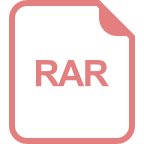





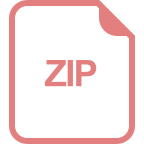