JAVA实现双向循环链表代码
时间: 2023-10-26 18:48:14 浏览: 86
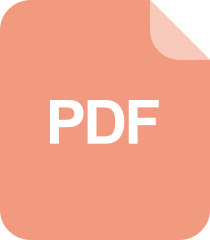
java双向循环链表的实现代码
Sure! Here's an example of Java code to implement a doubly circular linked list:
```java
public class DoublyCircularLinkedList {
private Node head;
private int size;
private class Node {
private int data;
private Node previous;
private Node next;
public Node(int data) {
this.data = data;
}
}
// Constructor
public DoublyCircularLinkedList() {
head = null;
size = 0;
}
// Check if the list is empty
public boolean isEmpty() {
return head == null;
}
// Get the size of the list
public int getSize() {
return size;
}
// Add an element to the beginning of the list
public void addFirst(int data) {
Node newNode = new Node(data);
if (isEmpty()) {
newNode.next = newNode;
newNode.previous = newNode;
head = newNode;
} else {
newNode.next = head;
newNode.previous = head.previous;
head.previous.next = newNode;
head.previous = newNode;
head = newNode;
}
size++;
}
// Add an element to the end of the list
public void addLast(int data) {
Node newNode = new Node(data);
if (isEmpty()) {
newNode.next = newNode;
newNode.previous = newNode;
head = newNode;
} else {
newNode.next = head;
newNode.previous = head.previous;
head.previous.next = newNode;
head.previous = newNode;
}
size++;
}
// Remove the first element from the list
public void removeFirst() {
if (isEmpty()) {
System.out.println("List is empty!");
return;
}
if (size == 1) {
head = null;
} else {
head.next.previous = head.previous;
head.previous.next = head.next;
head = head.next;
}
size--;
}
// Remove the last element from the list
public void removeLast() {
if (isEmpty()) {
System.out.println("List is empty!");
return;
}
if (size == 1) {
head = null;
} else {
head.previous.previous.next = head;
head.previous = head.previous.previous;
}
size--;
}
// Display the elements of the list
public void display() {
if (isEmpty()) {
System.out.println("List is empty!");
return;
}
Node current = head;
do {
System.out.print(current.data + " ");
current = current.next;
} while (current != head);
System.out.println();
}
// Main method to test the implementation
public static void main(String[] args) {
DoublyCircularLinkedList list = new DoublyCircularLinkedList();
list.addFirst(3);
list.addFirst(2);
list.addFirst(1);
list.addLast(4);
list.addLast(5);
System.out.println("Elements of the list: ");
list.display();
list.removeFirst();
list.removeLast();
System.out.println("Elements after removing first and last: ");
list.display();
}
}
```
This code creates a DoublyCircularLinkedList class with methods to add elements to the beginning and end, remove elements from the beginning and end, check if the list is empty, get the size of the list, and display the elements of the list. The main method is used to test the implementation by adding elements and then removing the first and last elements.
阅读全文
相关推荐
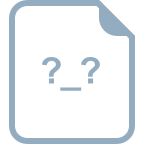
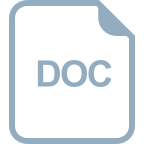
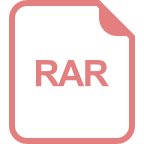
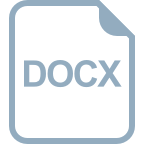
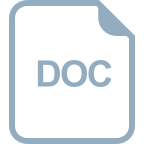



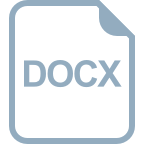
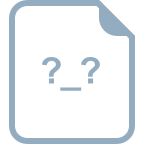
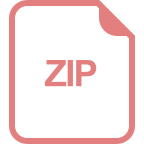
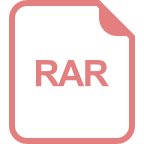


